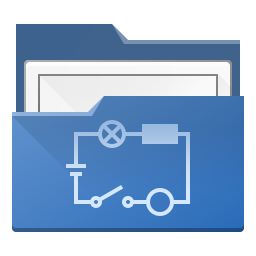 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
21 #include <QGraphicsView>
65 QString
title()
const;
80 bool event(QEvent *)
override;
125 void zoom(
const qreal zoom_factor);
131 void paste(
const QPointF & = QPointF(), QClipboard::Mode = QClipboard::Clipboard);
bool mustIntegrateTitleBlockTemplate(const TitleBlockTemplateLocation &) const
Definition: diagramview.cpp:896
virtual bool KeyReleaseEvent(QKeyEvent *event)
Definition: dveventinterface.cpp:78
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
void editSelection()
DiagramView::editSelection Edit the selected item if he can be edited and if only one item is selecte...
Definition: diagramview.cpp:1118
virtual bool mouseMoveEvent(QMouseEvent *event)
Definition: dveventinterface.cpp:44
void selectAll()
Definition: diagramview.cpp:122
static bool clipboardMayContainDiagram()
Diagram::clipboardMayContainDiagram.
Definition: diagram.cpp:2312
void applyReadOnly()
Definition: diagramview.cpp:908
void setVisualisationMode()
Definition: diagramview.cpp:270
The ChangeTitleBlockCommand class This command changes the title block properties for a particular di...
Definition: changetitleblockcommand.h:31
void setEventInterface(DiagramEventInterface *event_interface)
Diagram::setEventInterface Set event_interface has current interface. Diagram become the ownership of...
Definition: diagram.cpp:531
@ AnyConductor
Definition: diagramcontent.h:61
QColor color
Definition: conductorproperties.h:85
bool gestures() const
DiagramView::gestures.
Definition: diagramview.cpp:570
Definition: qetgraphicsitem.h:27
QString integrateTitleBlockTemplate(const TitleBlockTemplateLocation &, MoveTitleBlockTemplatesHandler *handler)
QETProject::integrateTitleBlockTemplate Integrate a title block template into this project.
Definition: qetproject.cpp:1065
Definition: integrationmovetemplateshandler.h:27
void scrollOnMovement(QKeyEvent *)
Definition: diagramview.cpp:746
void focusInEvent(QFocusEvent *) override
Definition: diagramview.cpp:637
void adjustSceneRect()
Diagram::adjustSceneRect Recalcul and adjust the size of the scene.
Definition: diagram.cpp:2166
bool fromXml(QDomDocument &, QPointF=QPointF(), bool=true, DiagramContent *=nullptr)
Diagram::fromXml Imports the described schema into an XML document. If a position is specified,...
Definition: diagram.cpp:1029
void modeChanged()
Signal emitted after the selection mode changed.
void adjustSceneRect()
DiagramView::adjustSceneRect Calcul and set the area of the scene visualized by this view.
Definition: diagramview.cpp:837
void editSelectedConductorColor()
DiagramView::editSelectedConductorColor Edit the color of the selected conductor; does nothing if mul...
Definition: diagramview.cpp:920
void changeLastTab()
change current diagramview to last tab
Definition: projectview.cpp:175
bool hasTextEditing()
DiagramContent::hasTextEditing.
Definition: diagramcontent.cpp:358
bool m_free_rubberbanding
Definition: diagramview.h:61
void editElementRequired(const ElementsLocation &)
Signal emitted when users wish to edit an element from the diagram.
void paste(const QPointF &=QPointF(), QClipboard::Mode=QClipboard::Clipboard)
DiagramView::paste Import the element stored in the clipboard to the diagram.
Definition: diagramview.cpp:366
void handleTitleBlockDrop(QDropEvent *)
DiagramView::handleTitleBlockDrop Handle the dropEvent that contain data of a titleblock.
Definition: diagramview.cpp:206
virtual bool mousePressEvent(QMouseEvent *event)
Definition: dveventinterface.cpp:39
virtual bool mouseReleaseEvent(QMouseEvent *event)
Definition: dveventinterface.cpp:49
void setEventInterface(DVEventInterface *event_interface)
DiagramView::setEventInterface Set an event interface to diagram view. If diagram view already have a...
Definition: diagramview.cpp:1140
void changeFirstTab()
change current diagramview to first tab
Definition: projectview.cpp:190
Definition: diagramcontent.h:46
The DiagramEventAddElement class This diagram event add a new element, for each left click button at ...
Definition: diagrameventaddelement.h:32
bool isEmpty() const
Diagram::isEmpty An empty schema contains no element, conductor, or text field.
Definition: diagram.cpp:680
The ResetConductorCommand class This command resets conductor paths.
Definition: diagramcommands.h:278
void changeTabDown()
change current diagramview to next folio
Definition: projectview.cpp:129
void dragEnterEvent(QDragEnterEvent *) override
Definition: diagramview.cpp:144
QRectF viewedSceneRect() const
Definition: diagramview.cpp:875
QETDiagramEditor * diagramEditor() const
Definition: diagramview.cpp:1228
void editConductorColor(Conductor *)
Definition: diagramview.cpp:936
QRectF rowsRect() const
BorderTitleBlock::rowsRect.
Definition: bordertitleblock.cpp:157
QList< Conductor * > conductors(int=AnyConductor) const
DiagramContent::conductors.
Definition: diagramcontent.cpp:167
void editDiagramProperties()
DiagramView::editDiagramProperties Edit the properties of the viewed digram.
Definition: diagramview.cpp:829
void mousePressEvent(QMouseEvent *) override
Definition: diagramview.cpp:398
QPointF m_drag_last_pos
Definition: diagramview.h:56
void resetConductors()
Definition: diagramview.cpp:973
BorderTitleBlock border_and_titleblock
Diagram dimensions and title block.
Definition: diagram.h:83
void selectNothing()
Definition: diagramview.cpp:129
QETProject * project() const
Diagram::project.
Definition: diagram.cpp:2326
void keyReleaseEvent(QKeyEvent *) override
Definition: diagramview.cpp:734
void loadCndFolioSeq()
Diagram::loadCndFolioSeq This class loads all conductor folio sequential variables related to the cur...
Definition: diagram.cpp:1942
bool isElement() const
ElementsLocation::isElement.
Definition: elementslocation.cpp:412
The Diagram class This class represents an electric diagram. It manages its various child elements,...
Definition: diagram.h:56
DiagramView(const DiagramView &)
void keyPressEvent(QKeyEvent *) override
DiagramView::keyPressEvent Handles "key press" events. Reimplemented here to switch to visualisation ...
Definition: diagramview.cpp:649
bool m_fresh_focus_in
Definition: diagramview.h:57
QETProject * parentProject() const
Definition: templatelocation.cpp:116
virtual bool switchToSelectionModeIfNeeded(QInputEvent *e)
Definition: diagramview.cpp:1076
Definition: titleblockproperties.h:29
virtual bool selectedItemHasFocus()
Definition: diagramview.cpp:1106
void paintEvent(QPaintEvent *event) override
DiagramView::paintEvent Reimplemented from QGraphicsView.
Definition: diagramview.cpp:1037
void selectInvert()
Definition: diagramview.cpp:136
virtual bool isCtrlShifting(QInputEvent *)
Definition: diagramview.cpp:1087
void pasteHere()
Definition: diagramview.cpp:390
void mouseDoubleClickEvent(QMouseEvent *) override
DiagramView::mouseDoubleClickEvent.
Definition: diagramview.cpp:1242
bool isValid() const
Definition: templatelocation.cpp:87
void setSelectionMode()
Definition: diagramview.cpp:280
Definition: templatelocation.h:29
TitleBlockProperties exportTitleBlock()
BorderTitleBlock::exportTitleBlock.
Definition: bordertitleblock.cpp:279
QHash< Qt::Corner, ConductorProfile > ConductorProfilesGroup
Definition: conductor.h:37
void cut()
Definition: diagramview.cpp:343
void handleTextDrop(QDropEvent *)
Definition: diagramview.cpp:255
The AddItemCommand class This command add an item in a diagram The item to add is template,...
Definition: diagramcommands.h:42
void mouseMoveEvent(QMouseEvent *) override
DiagramView::mouseMoveEvent Manage the event move mouse.
Definition: diagramview.cpp:455
DVEventInterface * m_event_interface
Definition: diagramview.h:52
void adjustGridToZoom()
Definition: diagramview.cpp:864
QPolygonF m_free_rubberband
Definition: diagramview.h:60
void zoom(const qreal zoom_factor)
DiagramView::zoom Zomm the view. A zoom_factor > 1 zoom in. A zoom_factor < 1 zoom out.
Definition: diagramview.cpp:294
static void create(Diagram *d, const QPolygonF &polygon)
ConductorCreator::create Create an electrical potential between the terminals of the diagram d,...
Definition: conductorcreator.cpp:77
void zoomReset()
Definition: diagramview.cpp:335
QUndoStack & undoStack()
Diagram::undoStack.
Definition: diagram.h:397
void freeRubberBandChanged(QPolygonF polygon)
void dropEvent(QDropEvent *) override
Definition: diagramview.cpp:169
QIcon QETLogo
Definition: qeticons.cpp:151
void finish()
finish emited when the interface finish is work
virtual bool keyPressEvent(QKeyEvent *event)
DVEventInterface::keyPressEvent By default, press escape key abort the curent action....
Definition: dveventinterface.cpp:66
int count(int=All) const
DiagramContent::count.
Definition: diagramcontent.cpp:401
static const qreal margin
margin around the diagram
Definition: diagram.h:97
QList< QAction * > m_separators
Definition: diagramview.h:59
void loadElmtFolioSeq()
Diagram::loadElmtFolioSeq This class loads all folio sequential variables related to the current auto...
Definition: diagram.cpp:1857
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
static void diagramPropertiesDialog(Diagram *diagram, QWidget *parent=nullptr)
DiagramPropertiesDialog::diagramPropertiesDialog Static method to get a DiagramPropertiesDialog.
Definition: diagrampropertiesdialog.cpp:129
void showDiagram(Diagram *)
Signal emmitted when diagram must be show.
QRectF titleBlockRect() const
BorderTitleBlock::titleBlockRect.
Definition: bordertitleblock.cpp:81
@ All
Definition: diagramcontent.h:65
Definition: qetproject.h:51
QRectF columnsRect() const
BorderTitleBlock::columnsRect.
Definition: bordertitleblock.cpp:143
void zoomContent()
Definition: diagramview.cpp:327
void updateWindowTitle()
Definition: diagramview.cpp:857
The ProjectView class This class provides a widget displaying the diagrams of a particular project us...
Definition: projectview.h:79
Diagram * diagram()
Definition: diagramview.h:67
void titleChanged(DiagramView *, const QString &)
Signal emitted after the diagram title changed.
@ SelectedOnly
Definition: diagramcontent.h:66
Definition: qetdiagrameditor.h:51
QAction * m_paste_here
Definition: diagramview.h:53
QString title() const
Definition: diagramview.cpp:814
void contextMenuEvent(QContextMenuEvent *) override
DiagramView::contextMenuEvent.
Definition: diagramview.cpp:1194
void mouseReleaseEvent(QMouseEvent *) override
DiagramView::mouseReleaseEvent Manage event release click mouse.
Definition: diagramview.cpp:516
void changeTabUp()
change current diagramview to previous tab
Definition: projectview.cpp:152
The BorderTitleBlock class This class represents the border and the titleblock which frame a particul...
Definition: bordertitleblock.h:37
QAction * m_multi_paste
Definition: diagramview.h:54
QList< DiagramTextItem * > selectedTexts() const
DiagramContent::selectedTexts.
Definition: diagramcontent.cpp:130
The ConductorProperties class This class represents the functional properties of a particular conduct...
Definition: conductorproperties.h:68
QIcon EditPaste
Definition: qeticons.cpp:67
Definition: multipastedialog.h:32
Definition: independenttextitem.h:28
void fromString(const QString &)
Definition: templatelocation.cpp:94
The CutDiagramCommand class This command cuts content from a particular diagram.
Definition: diagramcommands.h:118
The PasteDiagramCommand class This command pastes some content onto a particular diagram.
Definition: diagramcommands.h:88
QList< QGraphicsItem * > items(int=All) const
DiagramContent::items.
Definition: diagramcontent.cpp:374
QString template_name
Name of the template used to render the title block - an empty string means "the default template pro...
Definition: titleblockproperties.h:65
Diagram * m_diagram
Definition: diagramview.h:51
void handleElementDrop(QDropEvent *)
DiagramView::handleElementDrop Handle the drop of an element.
Definition: diagramview.cpp:185
bool gestureEvent(QGestureEvent *event)
DiagramView::gestureEvent Use the pinch of the trackpad for zoom.
Definition: diagramview.cpp:616
The DVEventInterface class This class is the main interface for manage event of a Diagram View....
Definition: dveventinterface.h:43
Definition: diagramview.h:38
void setDisplayGrid(bool)
Diagram::setDisplayGrid Set whether the diagram grid should be drawn.
Definition: diagram.h:333
void findElementRequired(const ElementsLocation &)
Signal emitted when users wish to locate an element from the diagram within elements collection.
Definition: conductor.h:43
DiagramView(Diagram *diagram, QWidget *=nullptr)
Definition: diagramview.cpp:54
~DiagramView() override
Definition: diagramview.cpp:116
void wheelEvent(QWheelEvent *) override
DiagramView::wheelEvent Manage wheel event of mouse.
Definition: diagramview.cpp:581
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
void zoomFit()
Definition: diagramview.cpp:318
void copy()
Definition: diagramview.cpp:353
virtual bool wheelEvent(QWheelEvent *event)
Definition: dveventinterface.cpp:54
QIcon tr
Definition: qeticons.cpp:206
QList< QAction * > contextMenuActions() const
DiagramView::contextMenuActions.
Definition: diagramview.cpp:1152
ProjectView * currentProjectView() const
Definition: qetdiagrameditor.cpp:1129
virtual bool switchToVisualisationModeIfNeeded(QInputEvent *e)
Definition: diagramview.cpp:1061
bool event(QEvent *) override
DiagramView::event Manage the event on this diagram view. -At first activation (QEvent::WindowActivat...
Definition: diagramview.cpp:1006
QString name() const
Definition: templatelocation.cpp:73
QPoint m_paste_here_pos
Definition: diagramview.h:55
void dragMoveEvent(QDragMoveEvent *) override
Definition: diagramview.cpp:160
bool m_first_activation
Definition: diagramview.h:58