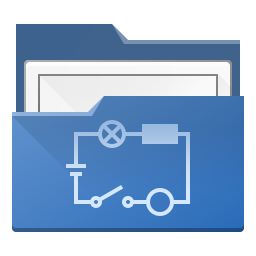 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTSCOLLECTIONWIDGET_H
19 #define ELEMENTSCOLLECTIONWIDGET_H
24 #include <QModelIndex>
76 void hideItem(
bool hide,
const QModelIndex &index = QModelIndex(),
bool recursive =
true);
77 void showAndExpandItem (
const QModelIndex &index,
bool parent =
true,
bool child =
false);
116 #endif // ELEMENTSCOLLECTIONWIDGET_H
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
static QETDiagramEditor * diagramEditorAncestorOf(const QWidget *child)
QETApp::diagramEditorAncestorOf.
Definition: qetapp.cpp:961
Definition: newelementwizard.h:39
void setUpData(ElementCollectionItem *eci)
Definition: elementcollectionitem.cpp:250
The ElementsTreeView class This class just reimplement startDrag from QTreeView, for set a custom pix...
Definition: elementstreeview.h:32
QIcon FolderOnlyThis
Definition: qeticons.cpp:98
void addLocation(const ElementsLocation &location)
ElementsCollectionModel::addLocation Add the element or directory to this model. If the location is a...
Definition: elementscollectionmodel.cpp:336
static QList< QETElementEditor * > elementEditors()
QETApp::elementEditors.
Definition: qetapp.cpp:1223
QIcon FolderEdit
Definition: qeticons.cpp:96
The QETApp class This class represents the QElectroTech application.
Definition: qetapp.h:55
QList< ElementCollectionItem * > directoriesChild() const
ElementCollectionItem::directoriesChild.
Definition: elementcollectionitem.cpp:221
QIcon FolderDelete
Definition: qeticons.cpp:95
void loadCollections(bool common_collection, bool custom_collection, QList< QETProject * > projects)
ElementsCollectionModel::loadCollections Load the several collections in this model....
Definition: elementscollectionmodel.cpp:259
void removeProject(QETProject *project)
ElementsCollectionModel::removeProject Remove project from this model.
Definition: elementscollectionmodel.cpp:424
QList< ElementCollectionItem * > elementsChild() const
ElementCollectionItem::elementsChild.
Definition: elementcollectionitem.cpp:207
ElementsLocation createdLocation() const
ElementsCategoryEditor::createdLocation.
Definition: elementscategoryeditor.cpp:97
virtual QString collectionPath() const =0
bool isDir() const override
FileElementCollectionItem::isDir.
Definition: fileelementcollectionitem.cpp:93
virtual void clearData()
ElementCollectionItem::clearData Reset the data.
Definition: elementcollectionitem.cpp:32
QList< QETProject * > project() const
ElementsCollectionModel::project.
Definition: elementscollectionmodel.cpp:455
@ Type
Definition: fileelementcollectionitem.h:34
The ElementCollectionItem class This class represent a item (a directory or an element) in a element ...
Definition: elementcollectionitem.h:30
bool isFileSystem() const
ElementsLocation::isFileSystem.
Definition: elementslocation.cpp:428
void loadingProgressValueChanged(int)
bool isElement() const
ElementsLocation::isElement.
Definition: elementslocation.cpp:412
The ElementsCategoryEditor class This class provides a dialog to edit an existing category or create ...
Definition: elementscategoryeditor.h:34
virtual bool isDir() const =0
QMessageBox::StandardButton question(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:53
QIcon ElementEdit
Definition: qeticons.cpp:85
QIcon FolderNew
Definition: qeticons.cpp:97
virtual QString localName()=0
QIcon FolderOpen
Definition: qeticons.cpp:99
QMessageBox::StandardButton information(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:38
bool isDirectory() const
ElementsLocation::isDirectory.
Definition: elementslocation.cpp:420
QIcon FolderProperties
Definition: qeticons.cpp:100
void preselectedLocation(const ElementsLocation &location)
NewElementWizard::preselectedLocation Select item in the tree view represented by location,...
Definition: newelementwizard.cpp:62
QIcon ElementNew
Definition: qeticons.cpp:86
The FileElementCollectionItem class This class specialise ElementCollectionItem for manage a collecti...
Definition: fileelementcollectionitem.h:30
@ Type
Definition: xmlprojectelementcollectionitem.h:36
QString fileSystemPath() const
ElementsLocation::fileSystemPath.
Definition: elementslocation.cpp:193
QIcon ElementDelete
Definition: qeticons.cpp:84
QIcon ViewRefresh
Definition: qeticons.cpp:173
void openElementLocations(const QList< ElementsLocation > &)
QETApp::openElementLocations Open the elements whose location is passed in parameter....
Definition: qetapp.cpp:1637
QString collectionPath(bool protocol=true) const
ElementsLocation::collectionPath Return the path of the represented element relative to collection if...
Definition: elementslocation.cpp:160
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
bool isCollectionRoot() const override
FileElementCollectionItem::isCollectionRoot.
Definition: fileelementcollectionitem.cpp:216
void loadingProgressRangeChanged(int, int)
static QETApp * instance()
QETApp::instance.
Definition: qetapp.cpp:153
Definition: qetproject.h:51
QModelIndex indexFromLocation(const ElementsLocation &location)
ElementsCollectionModel::indexFromLocation Return the index who represent location....
Definition: elementscollectionmodel.cpp:543
QString collectionPath() const override
FileElementCollectionItem::collectionPath.
Definition: fileelementcollectionitem.cpp:191
Definition: qetdiagrameditor.h:51
bool isCollectionRoot() const override
XmlProjectElementCollectionItem::isCollectionRoot.
Definition: xmlprojectelementcollectionitem.cpp:120
int type() const override
Definition: elementcollectionitem.h:35
QMessageBox::StandardButton warning(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:68
Definition: elementscollectionmodel.h:32
The XmlProjectElementCollectionItem class This class specialise ElementCollectionItem for manage an x...
Definition: xmlprojectelementcollectionitem.h:32
QIcon FolderShowAll
Definition: qeticons.cpp:101
void saveToLocation(ElementsLocation loc)
virtual bool isElement() const =0
QIcon tr
Definition: qeticons.cpp:206
bool isCommonCollection() const
FileElementCollectionItem::isCommonCollection.
Definition: fileelementcollectionitem.cpp:229
Definition: qetelementeditor.h:33
void highlightUnusedElement()
ElementsCollectionModel::highlightUnusedElement Highlight every unused element of managed project.
Definition: elementscollectionmodel.cpp:465