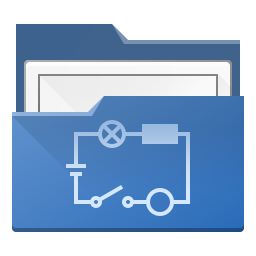 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENT_SCENE_H
19 #define ELEMENT_SCENE_H
104 virtual int xGrid()
const;
105 virtual int yGrid()
const;
106 virtual void setGrid(
int,
int);
107 virtual const QDomDocument
toXml(
bool =
true);
109 virtual void fromXml(
const QDomDocument &,
110 const QPointF & = QPointF(),
113 virtual void reset();
114 virtual QList<CustomElementPart *>
primitives()
const;
115 virtual QList<QGraphicsItem *>
180 Q_DECLARE_OPERATORS_FOR_FLAGS(ElementScene::ItemOptions)
188 m_names_list = nameslist;
void enableAnimation(bool animate=true)
QPropertyUndoCommand::enableAnimation True to enable animation.
Definition: qpropertyundocommand.cpp:92
@ NonSelected
Definition: elementscene.h:52
Behavior m_behavior
Definition: elementscene.h:76
QPointF snapToGrid(QPointF point)
ElementScene::snapToGrid Rounds the coordinates of the point passed as a parameter so that this point...
Definition: elementscene.cpp:1270
void setInformations(const QString &)
ElementScene::setInformations.
Definition: elementscene.h:211
Definition: elementprimitivedecorator.h:41
QRectF elementSceneGeometricRect() const
ElementScene::elementSceneGeometricRect.
Definition: elementscene.cpp:569
virtual void fromXml(const QDomDocument &, const QPointF &=QPointF(), bool=true, ElementContent *=nullptr)
ElementScene::fromXml Imports the element described in an XML document. If a position is specified,...
Definition: elementscene.cpp:537
@ Type
Definition: qetgraphicshandleritem.h:42
ElementContent loadContent(const QDomDocument &)
ElementScene::loadContent Create and load the content describe in the xml document.
Definition: elementscene.cpp:1113
void setNames(const NamesList &)
ElementScene::setNames.
Definition: elementscene.h:187
QList< QGraphicsItem * > ElementContent
Definition: elementcontent.h:21
void cut()
ElementScene::cut Handles the fact of cutting the selection = exporting it in XML to the clipboard th...
Definition: elementscene.cpp:648
Definition: elementview.h:27
void init()
ESEventInterface::init Init this event interface.
Definition: eseventinterface.cpp:38
virtual QList< QGraphicsItem * > zItems(ItemOptions options=ItemOptions(SortByZValue|IncludeTerminals|SelectedOrNot)) const
ElementScene::zItems.
Definition: elementscene.cpp:911
@ Type
Definition: parttext.h:52
QUndoStack & undoStack()
ElementScene::undoStack.
Definition: elementscene.cpp:603
void managePrimitivesGroups()
ElementScene::managePrimitivesGroups Ensure the decorator is adequately shown, hidden or updated so i...
Definition: elementscene.cpp:1318
void addPrimitive(QGraphicsItem *)
ElementScene::addPrimitive Add a primitive to the scene by wrapping it within an ElementPrimitiveDeco...
Definition: elementscene.cpp:1233
void fromXml(const QDomElement &, const QString &="property")
Definition: diagramcontext.cpp:157
void mouseDoubleClickEvent(QGraphicsSceneMouseEvent *event) override
ElementScene::mouseDoubleClickEvent.
Definition: elementscene.cpp:174
void slot_invertSelection()
ElementScene::slot_invertSelection Inverse Selection.
Definition: elementscene.cpp:753
QRectF m_defined_paste_area
Definition: elementscene.h:80
QIcon PartArc
Definition: qeticons.cpp:127
const QString version
QElectroTech version (as string, used to mark projects and elements XML documents)
Definition: qet.h:32
void partsAdded()
Signal emitted after one or several parts were added.
QString m_last_copied
Definition: elementscene.h:82
virtual bool isFinish() const
Definition: eseventinterface.cpp:106
QUndoStack m_undo_stack
Definition: elementscene.h:73
void contextMenuEvent(QGraphicsSceneContextMenuEvent *event) override
ElementScene::contextMenuEvent Display the context menu event, only if behavior are Normal.
Definition: elementscene.cpp:275
void partsZValueChanged()
Signal emitted when the zValue of one or several parts change.
QIcon PartText
Definition: qeticons.cpp:134
Definition: editorcommands.h:87
void stackAction(ElementEditionCommand *)
ElementScene::stackAction Push the provided command on the undo stack.
Definition: elementscene.cpp:1364
DiagramContext m_elmt_information
Definition: elementscene.h:71
virtual const QDomDocument toXml(bool=true)
ElementScene::toXml Export this element as a xml file.
Definition: elementscene.cpp:394
void setInformationText(const QString &text)
Definition: namelistdialog.cpp:42
void partsRemoved()
Signal emitted after one or several parts were removed.
void centerElementToOrigine()
ElementScene::centerElementToOrigine try to center better is possible the element to the scene (the c...
Definition: elementscene.cpp:1291
int m_x_grid
Definition: elementscene.h:87
QGIManager m_qgi_manager
element kind info
Definition: elementscene.h:72
virtual void reset()
ElementScene::reset Remove all QGraphicsItems in the scene and clear the undo stack.
Definition: elementscene.cpp:1006
static bool zValueLessThan(QGraphicsItem *, QGraphicsItem *)
ElementScene::zValueLessThan.
Definition: elementscene.cpp:1282
void slot_delete()
ElementScene::slot_delete Delete selected items.
Definition: elementscene.cpp:766
@ PasteArea
Definition: elementscene.h:46
virtual void fromXml(const QDomElement &)=0
virtual void setGrid(int, int)
ElementScene::setGrid.
Definition: elementscene.cpp:381
The ElementEditionCommand class ElementEditionCommand is the base class for all commands classes invo...
Definition: editorcommands.h:33
void pasteAreaDefined(const QRectF &)
Signal emitted when users have defined the copy/paste area.
ElementScene::Behavior behavior() const
Definition: elementscene.cpp:352
virtual int xGrid() const
ElementScene::xGrid.
Definition: elementscene.cpp:361
virtual ElementContent selectedContent() const
ElementScene::selectedContent.
Definition: elementscene.cpp:977
int m_y_grid
Definition: elementscene.h:88
~ElementScene() override
ElementScene::~ElementScene.
Definition: elementscene.cpp:79
The NameListDialog class Provide a dialog for let user define localized string;.
Definition: namelistdialog.h:34
virtual int yGrid() const
ElementScene::yGrid.
Definition: elementscene.cpp:370
void fromTextFieldXml(const QDomElement &dom_element)
PartDynamicTextField::fromTextFieldXml Setup this text from the xml definition of a text field (The x...
Definition: partdynamictextfield.cpp:225
QString m_elmt_type
Extra informations.
Definition: elementscene.h:69
QIcon PartRectangle
Definition: qeticons.cpp:132
Definition: editorcommands.h:265
QETElementEditor * editor() const
ElementScene::editor.
Definition: elementscene.cpp:683
void drawForeground(QPainter *, const QRectF &) override
ElementScene::drawForeground Draws the background of the editor, ie the hotspot indicator.
Definition: elementscene.cpp:294
ElementScene(const ElementScene &)
@ IncludeTerminals
Definition: elementscene.h:49
bool containsTerminals() const
ElementScene::containsTerminals.
Definition: elementscene.cpp:589
Definition: partterminal.h:30
The PartDynamicTextField class This class represents an editable dynamic text field which may be used...
Definition: partdynamictextfield.h:34
void mouseMoveEvent(QGraphicsSceneMouseEvent *) override
ElementScene::mouseMoveEvent.
Definition: elementscene.cpp:97
virtual QList< CustomElementPart * > primitives() const
ElementScene::primitives.
Definition: elementscene.cpp:895
QETElementEditor * m_element_editor
Definition: elementscene.h:77
Definition: editorcommands.h:65
void release(QGraphicsItem *)
Definition: qgimanager.cpp:60
void slot_editAuthorInformations()
ElementScene::slot_editAuthorInformations Starts a dialog to edit the additional information of this ...
Definition: elementscene.cpp:790
void setEventInterface(ESEventInterface *event_interface)
ElementScene::setEventInterface Set a new event interface.
Definition: elementscene.cpp:317
@ Type
Definition: elementprimitivedecorator.h:48
void mouseReleaseEvent(QGraphicsSceneMouseEvent *) override
ElementScene::mouseReleaseEvent.
Definition: elementscene.cpp:147
QString informations() const
ElementScene::informations.
Definition: elementscene.h:203
NamesList names() const
ElementScene::names.
Definition: elementscene.h:195
@ SortByZValue
Definition: elementscene.h:48
@ SelectedOrNot
Definition: elementscene.h:53
void slot_deselectAll()
ElementScene::slot_deselectAll deselect all item.
Definition: elementscene.cpp:744
Definition: editorcommands.h:149
QIcon PartEllipse
Definition: qeticons.cpp:129
void initPasteArea()
ElementScene::initPasteArea Initializes the paste area.
Definition: elementscene.cpp:1243
void slot_selectAll()
ElementScene::slot_selectAll Select all items.
Definition: elementscene.cpp:736
ESEventInterface * m_event_interface
Definition: elementscene.h:75
QGIManager & qgiManager()
ElementScene::qgiManager.
Definition: elementscene.cpp:612
NamesList m_names_list
Definition: elementscene.h:67
void needZoomFit()
Signal emitted when need zoomFit.
void elementInfoChanged()
DiagramContext elementInformation() const
Definition: elementscene.h:103
void slot_select(const ElementContent &)
ElementScene::slot_select Select the item in content, every others items in the scene are deselected.
Definition: elementscene.cpp:706
void clearEventInterface()
ElementScene::clearEventInterface Clear the current event interface.
Definition: elementscene.cpp:333
void fromXml(const QDomElement &, const QHash< QString, QString > &=QHash< QString, QString >())
Definition: nameslist.cpp:113
ItemOption
Definition: elementscene.h:47
QGraphicsRectItem * m_paste_area
Definition: elementscene.h:79
bool applyInformations(const QDomDocument &)
ElementScene::applyInformations Applies the information (dimensions, hostpot, orientations,...
Definition: elementscene.cpp:1065
Definition: nameslist.h:30
void mousePressEvent(QGraphicsSceneMouseEvent *) override
ElementScene::mousePressEvent.
Definition: elementscene.cpp:128
ElementContent addContentAtPos(const ElementContent &, const QPointF &)
ElementScene::addContentAtPos Add content content to this element.
Definition: elementscene.cpp:1205
QMutex * m_decorator_lock
Definition: elementscene.h:151
void setBehavior(ElementScene::Behavior)
ElementScene::setBehavior Modify the current behavior of this scene.
Definition: elementscene.cpp:348
void copy()
ElementScene::copy Handles the fact of copying the selection = exporting it as XML to the clipboard.
Definition: elementscene.cpp:662
static bool clipboardMayContainElement()
ElementScene::clipboardMayContainElement.
Definition: elementscene.cpp:621
QString elementType() const
Definition: elementscene.h:101
void slot_editProperties()
ElementScene::slot_editProperties Open dialog to edit the element properties.
Definition: elementscene.cpp:842
void toXml(QDomElement &, const QString &="property") const
Definition: diagramcontext.cpp:142
The CustomElementPart class This abstract class represents a primitive of the visual representation o...
Definition: customelementpart.h:40
Behavior
Definition: elementscene.h:46
Definition: qgimanager.h:27
friend class ChangePropertiesCommand
Definition: elementscene.h:41
QIcon PartLine
Definition: qeticons.cpp:130
virtual QRectF boundingRectFromXml(const QDomDocument &)
ElementScene::boundingRectFromXml.
Definition: elementscene.cpp:496
QString m_informations
List of localized names.
Definition: elementscene.h:68
void keyPressEvent(QKeyEvent *event) override
ElementScene::keyPressEvent manage key press event.
Definition: elementscene.cpp:194
Definition: parttext.h:30
bool wasCopiedFromThisElement(const QString &)
ElementScene::wasCopiedFromThisElement.
Definition: elementscene.cpp:637
QIcon PartPolygon
Definition: qeticons.cpp:131
@ Type
Definition: partdynamictextfield.h:67
The ElementScene class This class is the canvas allowing the visual edition of an electrial element....
Definition: elementscene.h:40
@ Normal
Definition: elementscene.h:46
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
ElementPrimitiveDecorator * m_decorator
Decorator item displayed when at least one item is selected.
Definition: elementscene.h:85
@ Selected
Definition: elementscene.h:51
DiagramContext elementKindInfo() const
Definition: elementscene.h:102
QDomElement toXml(QDomDocument &, const QHash< QString, QString > &=QHash< QString, QString >()) const
Definition: nameslist.cpp:169
DiagramContext m_elmt_kindInfo
element type
Definition: elementscene.h:70
ElementScene(QETElementEditor *, QObject *=nullptr)
ElementScene::ElementScene constructor.
Definition: elementscene.cpp:51
QIcon tr
Definition: qeticons.cpp:206
QRectF elementContentBoundingRect(const ElementContent &) const
ElementScene::elementContentBoundingRect.
Definition: elementscene.cpp:1039
@ IncludeHelperItems
Definition: elementscene.h:50
void slot_editNames()
ElementScene::slot_editNames Launch a dialog for edit the names of the edited element.
Definition: elementscene.cpp:864
@ AddPart
Definition: elementscene.h:46
QIcon Cancel
Definition: qeticons.cpp:34
Definition: qetelementeditor.h:33
Definition: diagramcontext.h:56
Definition: eseventinterface.h:30
NameListWidget * namelistWidget() const
NameListDialog::namelistWidget.
Definition: namelistdialog.cpp:51
ElementContent addContent(const ElementContent &)
ElementScene::addContent Add content content to this element.
Definition: elementscene.cpp:1185
void setElementInfo(const DiagramContext &dc)
ElementScene::setElementInfo.
Definition: elementscene.cpp:691
virtual void getPasteArea(const QRectF &)
ElementScene::getPasteArea.
Definition: elementscene.cpp:992