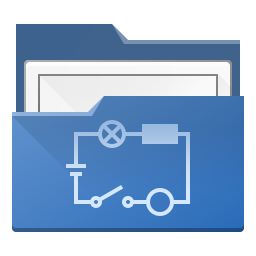 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef DELETEQGRAPHICSITEMCOMMAND_H
19 #define DELETEQGRAPHICSITEMCOMMAND_H
21 #include <QUndoCommand>
58 #endif // DELETEQGRAPHICSITEMCOMMAND_H
void undo() override
DeleteQGraphicsItemCommand::undo Undo this command.
Definition: deleteqgraphicsitemcommand.cpp:244
QList< Conductor * > conductors() const
Terminal::conductors.
Definition: terminal.cpp:715
DeleteQGraphicsItemCommand(const DeleteQGraphicsItemCommand &)
@ AnyConductor
Definition: diagramcontent.h:61
bool addTextToGroup(DynamicElementTextItem *text, ElementTextItemGroup *group)
Element::addTextToGroup Add the text text to the group group; If group isn't owned by this element re...
Definition: element.cpp:1432
The DynamicElementTextItem class This class provide a simple text field of element who can be added o...
Definition: dynamicelementtextitem.h:39
void setProperties(const ConductorProperties &property)
Conductor::setProperties Set property as current property of conductor.
Definition: conductor.cpp:1538
QList< Element * > linkedElements()
Element::linkedElements.
Definition: element.h:258
The QetGraphicsTableItem class This item display a table destined to represent the content of a QAbst...
Definition: qetgraphicstableitem.h:43
QList< QPair< Terminal *, Terminal * > > m_connected_terminals
Keep the parent group of each deleted element text item.
Definition: deleteqgraphicsitemcommand.h:53
Element * parentElement() const
ElementTextItemGroup::parentElement.
Definition: elementtextitemgroup.cpp:357
Definition: deleteqgraphicsitemcommand.h:31
void showMe()
Definition: diagram.h:161
virtual void addItem(QGraphicsItem *item)
Diagram::addItem Réimplemented from QGraphicsScene::addItem(QGraphicsItem *item) Do some specific ope...
Definition: diagram.cpp:1550
Diagram * diagram() const
Definition: conductor.cpp:559
Definition: diagramcontent.h:46
QList< Element * > m_elements
Definition: diagramcontent.h:69
Element * parentElement() const
Terminal::parentElement.
Definition: terminal.cpp:822
DeleteQGraphicsItemCommand(Diagram *diagram, const DiagramContent &content, QUndoCommand *parent=nullptr)
DeleteQGraphicsItemCommand::DeleteQGraphicsItemCommand.
Definition: deleteqgraphicsitemcommand.cpp:37
QList< Conductor * > conductors(int=AnyConductor) const
DiagramContent::conductors.
Definition: diagramcontent.cpp:167
QString sentence(int=All) const
DiagramContent::sentence.
Definition: diagramcontent.cpp:438
QHash< DynamicElementTextItem *, ElementTextItemGroup * > m_grp_texts_hash
Keep the parent element of each deleted dynamic element text item.
Definition: deleteqgraphicsitemcommand.h:52
void redo() override
DeleteQGraphicsItemCommand::redo Redo the delete command.
Definition: deleteqgraphicsitemcommand.cpp:282
The Diagram class This class represents an electric diagram. It manages its various child elements,...
Definition: diagram.h:56
bool removeTextFromGroup(DynamicElementTextItem *text, ElementTextItemGroup *group)
Element::removeTextFromGroup Remove the text text from the group group, en reparent text to this elem...
Definition: element.cpp:1455
QHash< QetGraphicsTableItem *, QPointer< QGraphicsScene > > m_table_scene_hash
Definition: deleteqgraphicsitemcommand.h:54
Diagram * m_diagram
Definition: deleteqgraphicsitemcommand.h:49
void setPotentialsOfRemovedElements()
DeleteQGraphicsItemCommand::setPotentialsOfRemovedElements This function creates new conductors (if n...
Definition: deleteqgraphicsitemcommand.cpp:121
QIcon Conductor
Definition: qeticons.cpp:35
virtual void removeItem(QGraphicsItem *item)
Diagram::removeItem Reimplemented from QGraphicsScene::removeItem(QGraphicsItem *item) Do some specif...
Definition: diagram.cpp:1581
QHash< DynamicElementTextItem *, Element * > m_elmt_text_hash
keep linked element for each removed element linked to other element.
Definition: deleteqgraphicsitemcommand.h:51
QSet< ElementTextItemGroup * > m_texts_groups
Definition: diagramcontent.h:78
void removeDynamicTextItem(DynamicElementTextItem *deti)
Element::removeDynamicTextItem Remove deti, no matter if is a child of this element or a child of a g...
Definition: element.cpp:1287
The AddItemCommand class This command add an item in a diagram The item to add is template,...
Definition: diagramcommands.h:42
void release(QGraphicsItem *)
Definition: qgimanager.cpp:60
~DeleteQGraphicsItemCommand() override
Definition: deleteqgraphicsitemcommand.cpp:112
void addDynamicTextItem(DynamicElementTextItem *deti=nullptr)
Element::addDynamiqueTextItem Add deti as a dynamic text item of this element, deti is reparented to ...
Definition: element.cpp:1264
QVector< QetGraphicsTableItem * > m_tables
Definition: diagramcontent.h:80
The RemoveTextsGroupCommand class Manage the removinf of a texts group.
Definition: addelementtextcommand.h:85
QList< Terminal * > terminals() const
Element::terminals.
Definition: element.cpp:136
Element * parentElement() const
DynamicElementTextItem::ParentElement.
Definition: dynamicelementtextitem.cpp:227
QGIManager & qgiManager()
Diagram::qgiManager.
Definition: diagram.h:405
@ All
Definition: diagramcontent.h:65
QSet< DynamicElementTextItem * > m_element_texts
Definition: diagramcontent.h:77
The ElementTextItemGroup class This class represent a group of element text Texts in the group can be...
Definition: elementtextitemgroup.h:36
DiagramContent m_removed_contents
Definition: deleteqgraphicsitemcommand.h:48
The ConductorProperties class This class represents the functional properties of a particular conduct...
Definition: conductorproperties.h:68
QHash< Element *, QList< Element * > > m_link_hash
Definition: deleteqgraphicsitemcommand.h:50
QList< QGraphicsItem * > items(int=All) const
DiagramContent::items.
Definition: diagramcontent.cpp:374
ElementTextItemGroup * parentGroup() const
DynamicElementTextItem::parentGroup.
Definition: dynamicelementtextitem.cpp:236
bool m_remove_linked_table
Definition: deleteqgraphicsitemcommand.h:55
void manage(QGraphicsItem *)
Definition: qgimanager.cpp:48
Terminal * terminalInSamePotential(Terminal *terminal, Conductor *conductor_to_exclude)
DeleteQGraphicsItemCommand::terminalInSamePotential Return a terminal at the same potential of termin...
Definition: deleteqgraphicsitemcommand.cpp:214
Definition: conductor.h:43
The Terminal class This class represents a terminal of an electrical element, i.e....
Definition: terminal.h:35
@ Elements
Definition: diagramcontent.h:54
QIcon tr
Definition: qeticons.cpp:206