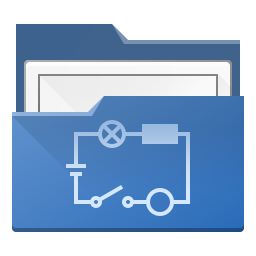 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELLIPSE_EDITOR_H
19 #define ELLIPSE_EDITOR_H
45 QDoubleSpinBox *
x, *
y, *
h, *
v;
52 bool setParts(QList <CustomElementPart *> parts)
override;
QList< CustomElementPart * > currentParts() const override
Definition: styleeditor.cpp:543
QList< CustomElementPart * > currentParts() const override
Definition: ellipseeditor.cpp:133
void activeConnections(bool)
Definition: ellipseeditor.cpp:252
QUndoStack & undoStack()
ElementScene::undoStack.
Definition: elementscene.cpp:603
bool m_locked
Definition: ellipseeditor.h:46
void editingFinishedV()
Definition: ellipseeditor.cpp:210
EllipseEditor(QETElementEditor *, PartEllipse *=nullptr, QWidget *=nullptr)
Definition: ellipseeditor.cpp:30
PartEllipse * part
Definition: ellipseeditor.h:43
EllipseEditor(const EllipseEditor &)
QDoubleSpinBox * v
Definition: ellipseeditor.h:45
void editingFinishedH()
Definition: ellipseeditor.cpp:187
void updateForm() override
Definition: ellipseeditor.cpp:236
void setUpChangeConnections()
setUpChangeConnections Setup the connection from the ellipse(s) to the widget, to update it when the ...
Definition: ellipseeditor.cpp:73
Definition: styleeditor.h:35
~EllipseEditor() override
Destructeur.
Definition: ellipseeditor.cpp:70
Definition: elementitemeditor.h:34
virtual ElementScene * elementScene() const
Definition: elementitemeditor.cpp:39
The PartEllipse class This class represents an ellipse primitive which may be used to compose the dra...
Definition: partellipse.h:31
void undo() override
PastePartsCommand::undo.
Definition: pastepartscommand.cpp:60
QDoubleSpinBox * x
Definition: ellipseeditor.h:45
bool setParts(QList< CustomElementPart * > parts) override
Definition: ellipseeditor.cpp:116
QList< QMetaObject::Connection > m_change_connections
Definition: ellipseeditor.h:47
StyleEditor * style_
Definition: ellipseeditor.h:44
bool setParts(QList< CustomElementPart * >) override
StyleEditor::setParts Set several parts to edit by this editor. Note : editor can accept or refuse to...
Definition: styleeditor.cpp:510
QDoubleSpinBox * y
Definition: ellipseeditor.h:45
CustomElementPart * currentPart() const override
Definition: ellipseeditor.cpp:129
The CustomElementPart class This abstract class represents a primitive of the visual representation o...
Definition: customelementpart.h:40
void editingFinishedY()
Definition: ellipseeditor.cpp:161
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
Definition: ellipseeditor.h:31
QDoubleSpinBox * h
Definition: ellipseeditor.h:45
void editingFinishedX()
Definition: ellipseeditor.cpp:137
QIcon tr
Definition: qeticons.cpp:206
void disconnectChangeConnections()
Definition: ellipseeditor.cpp:78
Definition: qetelementeditor.h:33
bool setPart(CustomElementPart *) override
Definition: ellipseeditor.cpp:94
QVariant property(const char *name) const override
Definition: customelementgraphicpart.h:297