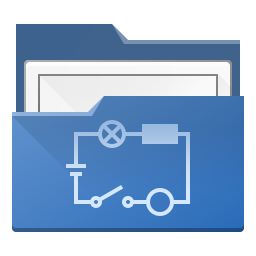 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTSCOLLECTIONMODEL2_H
19 #define ELEMENTSCOLLECTIONMODEL2_H
21 #include <QStandardItemModel>
38 QVariant
data(
const QModelIndex &index,
int role)
const override;
39 QMimeData *
mimeData(
const QModelIndexList &indexes)
const override;
41 bool canDropMimeData(
const QMimeData *
data, Qt::DropAction action,
int row,
int column,
const QModelIndex &parent)
const override;
42 bool dropMimeData(
const QMimeData *
data, Qt::DropAction action,
int row,
int column,
const QModelIndex &parent)
override;
44 void loadCollections(
bool common_collection,
bool custom_collection, QList<QETProject *> projects);
52 QList<QETProject *>
project()
const;
56 QList <ElementCollectionItem *>
items()
const;
80 #endif // ELEMENTSCOLLECTIONMODEL2_H
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
void itemRemovedFromCollection(const QString &path)
ElementsCollectionModel::itemRemovedFromCollection This method must be called by a signal,...
Definition: elementscollectionmodel.cpp:618
QList< ElementCollectionItem * > items() const
ElementCollectionItem::items.
Definition: elementcollectionitem.cpp:237
void setUpData(ElementCollectionItem *eci)
Definition: elementcollectionitem.cpp:250
bool setRootPath(const QString &path, bool set_data=true, bool hide_element=false)
FileElementCollectionItem::setRootPath Set path has root path for this file item. Use this function o...
Definition: fileelementcollectionitem.cpp:42
void addLocation(const ElementsLocation &location)
ElementsCollectionModel::addLocation Add the element or directory to this model. If the location is a...
Definition: elementscollectionmodel.cpp:336
void updateItem(const QString &path)
ElementsCollectionModel::updateItem Update the item at path.
Definition: elementscollectionmodel.cpp:647
XmlElementCollection * embeddedElementCollection() const
QETProject::embeddedCollection.
Definition: qetproject.cpp:236
ElementsCollectionModel(QObject *parent=Q_NULLPTR)
ElementsCollectionModel::ElementsCollectionModel Constructor.
Definition: elementscollectionmodel.cpp:35
ElementsLocation copy(ElementsLocation &source, ElementsLocation &destination)
ElementCollectionHandler::copy Copy the content of collection represented by source to the collection...
Definition: elementcollectionhandler.cpp:321
bool isElement() const override
XmlProjectElementCollectionItem::isElement.
Definition: xmlprojectelementcollectionitem.cpp:44
QString fileName() const
ElementLocation::fileName.
Definition: elementslocation.cpp:793
void loadCollections(bool common_collection, bool custom_collection, QList< QETProject * > projects)
ElementsCollectionModel::loadCollections Load the several collections in this model....
Definition: elementscollectionmodel.cpp:259
void removeProject(QETProject *project)
ElementsCollectionModel::removeProject Remove project from this model.
Definition: elementscollectionmodel.cpp:424
QList< ElementCollectionItem * > items() const
ElementsCollectionModel::items.
Definition: elementscollectionmodel.cpp:490
ElementCollectionItem * lastItemForPath(const QString &path, QString &no_found_path)
ElementCollectionItem::lastItemForPath Return the last existing item in this ElementCollectionItem hi...
Definition: elementcollectionitem.cpp:52
QList< ElementCollectionItem * > projectItems(QETProject *project) const
ElementsCollectionModel::projectItems.
Definition: elementscollectionmodel.cpp:508
virtual QString collectionPath() const =0
The XmlElementCollection class This class represent a collection of elements stored to xml.
Definition: xmlelementcollection.h:34
QString collectionPath() const override
XmlProjectElementCollectionItem::collectionPath.
Definition: xmlprojectelementcollectionitem.cpp:86
virtual void clearData()
ElementCollectionItem::clearData Reset the data.
Definition: elementcollectionitem.cpp:32
void addProject(QETProject *project, bool set_data=true)
ElementsCollectionModel::addProject Add project to this model.
Definition: elementscollectionmodel.cpp:391
QList< QETProject * > project() const
ElementsCollectionModel::project.
Definition: elementscollectionmodel.cpp:455
@ Type
Definition: fileelementcollectionitem.h:34
The ElementCollectionItem class This class represent a item (a directory or an element) in a element ...
Definition: elementcollectionitem.h:30
void elementAdded(QString collection_path)
elementAdded This signal is emited when a element is added to this collection
virtual void addChildAtPath(const QString &collection_name)=0
QList< ElementCollectionItem * > m_items_list_to_setUp
Definition: elementscollectionmodel.h:77
void loadingProgressValueChanged(int)
QFuture< void > m_future
Definition: elementscollectionmodel.h:76
The ElementCollectionHandler class Provide several method to copy element or directory from a collect...
Definition: elementcollectionhandler.h:109
int type() const override
Definition: fileelementcollectionitem.h:35
virtual void setUpData()=0
void elementIntegratedToCollection(const QString &path)
ElementsCollectionModel::elementIntegratedToCollection When an element is added to embedded collectio...
Definition: elementscollectionmodel.cpp:583
QString fileSystemPath() const
FileElementCollectionItem::fileSystemPath.
Definition: fileelementcollectionitem.cpp:61
void addCustomCollection(bool set_data=true)
ElementsCollectionModel::addCustomCollection Add the custom elements collection to this model.
Definition: elementscollectionmodel.cpp:316
QMimeData * mimeData(const QModelIndexList &indexes) const override
ElementsCollectionModel::mimeData Reimplemented from QStandardItemModel.
Definition: elementscollectionmodel.cpp:67
bool m_hide_element
Definition: elementscollectionmodel.h:75
void setUpData() override
XmlProjectElementCollectionItem::setUpData SetUp the data of this item.
Definition: xmlprojectelementcollectionitem.cpp:191
void directoryRemoved(QString collection_path)
directoryRemoved This signal is emited when a directory is removed to this collection
void hideElement()
ElementsCollectionModel::hideElement Hide element in this model, only directory is managed.
Definition: elementscollectionmodel.cpp:526
bool isHideElement()
Definition: elementscollectionmodel.h:59
ElementCollectionItem * itemAtPath(const QString &path)
ElementCollectionItem::itemAtPath.
Definition: elementcollectionitem.cpp:151
The FileElementCollectionItem class This class specialise ElementCollectionItem for manage a collecti...
Definition: fileelementcollectionitem.h:30
@ Type
Definition: xmlprojectelementcollectionitem.h:36
void addCommonCollection(bool set_data=true)
ElementsCollectionModel::addCommonCollection Add the common elements collection to this model.
Definition: elementscollectionmodel.cpp:297
void elementRemoved(QString collection_path)
elementRemoved This signal is emited when an element is removed to this collection
static QString customElementsDirN()
QETApp::customElementsDirN like QString QETApp::customElementsDir but without "/" at the end.
Definition: qetapp.cpp:705
bool isProject() const
ElementsLocation::isProject.
Definition: elementslocation.cpp:459
QString collectionPath(bool protocol=true) const
ElementsLocation::collectionPath Return the path of the represented element relative to collection if...
Definition: elementslocation.cpp:160
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
bool isCommonCollection() const
ElementsLocation::isCommonCollection.
Definition: elementslocation.cpp:440
void loadingProgressRangeChanged(int, int)
Definition: qetproject.h:51
static QString commonElementsDirN()
QETApp::commonElementsDirN like QString QETApp::commonElementsDir but without "/" at the end.
Definition: qetapp.cpp:693
QModelIndex indexFromLocation(const ElementsLocation &location)
ElementsCollectionModel::indexFromLocation Return the index who represent location....
Definition: elementscollectionmodel.cpp:543
void setUpData() override
FileElementCollectionItem::setUpData SetUp the data of this item.
Definition: fileelementcollectionitem.cpp:264
void setProject(QETProject *project, bool set_data=true, bool hide_element=false)
XmlProjectElementCollectionItem::setProject Set the project for this item. Use this method for set th...
Definition: xmlprojectelementcollectionitem.cpp:175
QString collectionPath() const override
FileElementCollectionItem::collectionPath.
Definition: fileelementcollectionitem.cpp:191
QVariant data(const QModelIndex &index, int role) const override
ElementsCollectionModel::data Reimplemented from QStandardItemModel.
Definition: elementscollectionmodel.cpp:47
int type() const override
Definition: elementcollectionitem.h:35
bool isCustomCollection() const
FileElementCollectionItem::isCustomCollection.
Definition: fileelementcollectionitem.cpp:238
Definition: elementscollectionmodel.h:32
The XmlProjectElementCollectionItem class This class specialise ElementCollectionItem for manage an x...
Definition: xmlprojectelementcollectionitem.h:32
bool isElement() const override
FileElementCollectionItem::isElement.
Definition: fileelementcollectionitem.cpp:106
QStringList mimeTypes() const override
ElementsCollectionModel::mimeTypes Reimplemented from QStandardItemModel.
Definition: elementscollectionmodel.cpp:94
QETProject * project() const
ElementsLocation::project.
Definition: elementslocation.cpp:365
virtual bool isElement() const =0
int type() const override
Definition: xmlprojectelementcollectionitem.h:37
void elementChanged(QString collection_path)
elementChanged This signal is emited when the defintion of the element at path was changed
bool isCustomCollection() const
ElementsLocation::isCustomCollection.
Definition: elementslocation.cpp:450
void addChildAtPath(const QString &collection_name) override
FileElementCollectionItem::addChildAtPath Ask to this item item to add a child with collection name c...
Definition: fileelementcollectionitem.cpp:249
QList< QETProject * > m_project_list
Definition: elementscollectionmodel.h:73
QHash< QETProject *, XmlProjectElementCollectionItem * > m_project_hash
Definition: elementscollectionmodel.h:74
bool isCommonCollection() const
FileElementCollectionItem::isCommonCollection.
Definition: fileelementcollectionitem.cpp:229
bool canDropMimeData(const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const override
ElementsCollectionModel::canDropMimeData Reimplemented from QStandardItemModel.
Definition: elementscollectionmodel.cpp:112
void highlightUnusedElement()
ElementsCollectionModel::highlightUnusedElement Highlight every unused element of managed project.
Definition: elementscollectionmodel.cpp:465
bool dropMimeData(const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) override
ElementsCollectionModel::dropMimeData Reimplemented from QStandardItemModel.
Definition: elementscollectionmodel.cpp:163
void setUpIcon() override
XmlProjectElementCollectionItem::setUpIcon SetUp the icon of this item. Because icon use several memo...
Definition: xmlprojectelementcollectionitem.cpp:223