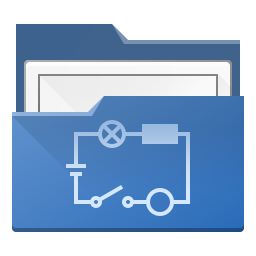 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef NUMEROTATIONCONTEXT_H
19 #define NUMEROTATIONCONTEXT_H
21 #include <QStringList>
23 #include <QDomElement>
37 const QVariant & = QVariant(1),
44 QStringList
itemAt(
const int)
const;
49 QDomElement
toXml(QDomDocument &,
const QString&);
57 #endif // NUMEROTATIONCONTEXT_H
QDomElement toXml(QDomDocument &, const QString &)
NumerotationContext::toXml Save the numerotation context in a QDomElement under the element name str.
Definition: numerotationcontext.cpp:150
QStringList content_
Definition: numerotationcontext.h:54
bool isEmpty() const
NumerotationContext::isEmpty.
Definition: numerotationcontext.cpp:101
void replaceValue(int, QString)
NumerotationContext::replaceValue This class replaces the current NC field value with content.
Definition: numerotationcontext.cpp:183
QString validRegExpNumber() const
NumerotationContext::validRegExpNumber.
Definition: numerotationcontext.cpp:126
bool keyIsNumber(const QString &) const
NumerotationContext::keyIsNumber.
Definition: numerotationcontext.cpp:142
Definition: numerotationcontext.h:31
QList< QDomElement > findInDomElement(const QDomElement &, const QString &)
Definition: qet.cpp:333
int size() const
NumerotationContext::size.
Definition: numerotationcontext.cpp:93
void operator<<(const NumerotationContext &)
NumerotationContext::operator << , append other.
Definition: numerotationcontext.cpp:85
NumerotationContext()
Definition: numerotationcontext.cpp:26
void clear()
NumerotationContext::clear, clear the content.
Definition: numerotationcontext.cpp:39
bool addValue(const QString &, const QVariant &=QVariant(1), const int=1, const int=0)
NumerotationContext::addValue add a new value on the contexte.
Definition: numerotationcontext.cpp:52
QStringList itemAt(const int) const
NumerotationContext::itemAt.
Definition: numerotationcontext.cpp:110
QString operator[](const int &) const
NumerotationContext::operator [].
Definition: numerotationcontext.cpp:78
QString validRegExpNum() const
validRegExpNum
Definition: numerotationcontext.cpp:118
bool keyIsAcceptable(const QString &) const
NumerotationContext::keyIsAcceptable.
Definition: numerotationcontext.cpp:134
void fromXml(QDomElement &)
NumerotationContext::fromXml load numerotation context from e.
Definition: numerotationcontext.cpp:172