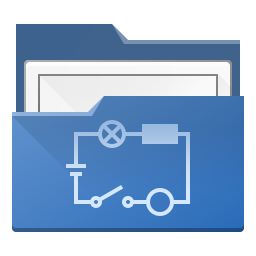 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef EDITOR_COMMANDS_H
19 #define EDITOR_COMMANDS_H
38 QUndoCommand * =
nullptr);
42 QUndoCommand * =
nullptr);
109 void undo()
override;
110 void redo()
override;
135 void undo()
override;
136 void redo()
override;
159 void undo()
override;
160 void redo()
override;
185 void undo()
override;
186 void redo()
override;
190 void applyRaise(
const QList<QGraphicsItem *> &);
191 void applyLower(
const QList<QGraphicsItem *> &);
217 void undo()
override;
218 void redo()
override;
241 void undo()
override;
242 void redo()
override;
250 void scale(
const QRectF &before,
const QRectF &after);
272 QUndoCommand *parent=
nullptr);
275 void undo()
override;
276 void redo()
override;
ElementEditionCommand(const ElementEditionCommand &)
ChangeNamesCommand(ElementScene *, const NamesList &, const NamesList &, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:252
DeletePartsCommand(const DeletePartsCommand &)
void undo() override
Definition: editorcommands.cpp:458
Definition: elementview.h:27
QGraphicsItem * part
Added primitive.
Definition: editorcommands.h:141
ElementView * elementView() const
Definition: editorcommands.cpp:79
AddPartCommand(const QString &, ElementScene *, QGraphicsItem *, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:204
QList< QGraphicsItem * > deleted_parts
Deleted primitives.
Definition: editorcommands.h:81
QHash< QGraphicsItem *, qreal > undo_hash
associates impacted primitives with their former zValues
Definition: editorcommands.h:197
void undo() override
Annule l'ajout.
Definition: editorcommands.cpp:223
void setElementView(ElementView *)
Definition: editorcommands.cpp:86
QList< QString > m_type
Definition: editorcommands.h:279
MovePartsCommand(const QPointF &, ElementScene *, const QList< QGraphicsItem * > &, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:164
NamesList names_before
List of former names.
Definition: editorcommands.h:165
@ Raise
Raise item one layer above their current one; zValues are incremented.
Definition: qet.h:47
bool first_redo
Prevent the first call to redo()
Definition: editorcommands.h:262
void redo() override
Supprime les parties.
Definition: editorcommands.cpp:127
QList< CustomElementPart * > scaled_primitives_
List of moved primitives.
Definition: editorcommands.h:256
void redo() override
Definition: editorcommands.cpp:574
void undo() override
Definition: editorcommands.cpp:567
Definition: editorcommands.h:87
DiagramContext m_elmt_information
Definition: elementscene.h:71
QET::DepthOption m_option
kind of treatment to apply
Definition: editorcommands.h:201
QList< DiagramContext > m_elmt_info
Definition: editorcommands.h:281
MovePartsCommand(const MovePartsCommand &)
void adjustText()
Definition: editorcommands.cpp:535
~ChangePropertiesCommand() override
Definition: editorcommands.cpp:565
The ElementEditionCommand class ElementEditionCommand is the base class for all commands classes invo...
Definition: editorcommands.h:33
Definition: editorcommands.h:175
~ChangeZValueCommand() override
Destructeur.
Definition: editorcommands.cpp:315
void redo() override
Refait le changement.
Definition: editorcommands.cpp:274
void applyRaise(const QList< QGraphicsItem * > &)
Definition: editorcommands.cpp:350
@ SendBackward
Send item to the background so they have the lowest zValue.
Definition: qet.h:49
ScalePartsCommand(const ScalePartsCommand &)
ElementScene * elementScene() const
Definition: editorcommands.cpp:476
@ BringForward
Bring item to the foreground so they have the highest zValue.
Definition: qet.h:46
QHash< QGraphicsItem *, qreal > redo_hash
associates impacted primitives with their new zValues
Definition: editorcommands.h:199
bool first_redo
Prevent the first call to redo()
Definition: editorcommands.h:143
QString m_elmt_type
Extra informations.
Definition: elementscene.h:69
Definition: editorcommands.h:265
bool first_redo
Prevent the first call to redo()
Definition: editorcommands.h:119
QList< DiagramContext > m_kind_info
Definition: editorcommands.h:280
ChangeNamesCommand(const ChangeNamesCommand &)
ElementView * m_view
Definition: editorcommands.h:58
~DeletePartsCommand() override
Destructeur : detruit egalement les parties supprimees.
Definition: editorcommands.cpp:111
CutPartsCommand(const CutPartsCommand &)
void undo() override
Restaure les parties supprimees.
Definition: editorcommands.cpp:118
ChangeZValueCommand(const ChangeZValueCommand &)
QList< QGraphicsItem * > moved_parts
List of moved primitives.
Definition: editorcommands.h:115
Definition: editorcommands.h:65
DepthOption
List the various kind of changes for the zValue.
Definition: qet.h:45
~ScalePartsCommand() override
Definition: editorcommands.cpp:452
AddPartCommand(const AddPartCommand &)
@ SortByZValue
Definition: elementscene.h:48
@ SelectedOrNot
Definition: elementscene.h:53
~AddPartCommand() override
Destructeur.
Definition: editorcommands.cpp:218
~MovePartsCommand() override
Destructeur.
Definition: editorcommands.cpp:178
Definition: editorcommands.h:149
ElementEditionCommand(ElementScene *=nullptr, ElementView *=nullptr, QUndoCommand *=nullptr)
ElementEditionCommand::ElementEditionCommand Constructs an ElementEditionCommand, thus embedding the ...
Definition: editorcommands.cpp:28
DeletePartsCommand(ElementScene *, const QList< QGraphicsItem * > &, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:97
@ Lower
Send item one layer below their current one; zValues are decremented.
Definition: qet.h:48
void applyBringForward(const QList< QGraphicsItem * > &)
Definition: editorcommands.cpp:332
CutPartsCommand(ElementScene *, const QList< QGraphicsItem * > &, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:142
ChangePropertiesCommand(ElementScene *scene, const QString &type, const DiagramContext &info, const DiagramContext &elmt_info, QUndoCommand *parent=nullptr)
ChangePropertiesCommand::ChangePropertiesCommand Change the properties of the drawed element.
Definition: editorcommands.cpp:551
Definition: editorcommands.h:99
QPair< QRectF, QRectF > transformation()
Definition: editorcommands.cpp:514
void setScaledPrimitives(const QList< CustomElementPart * > &)
Definition: editorcommands.cpp:483
QRectF original_rect_
original rect items fit in
Definition: editorcommands.h:258
ElementScene * elementScene() const
Definition: editorcommands.cpp:65
Definition: nameslist.h:30
void undo() override
Annule le deplacement.
Definition: editorcommands.cpp:182
ChangeZValueCommand(ElementScene *, QET::DepthOption, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:284
QList< CustomElementPart * > scaledPrimitives() const
Definition: editorcommands.cpp:491
void redo() override
Definition: editorcommands.cpp:465
~CutPartsCommand() override
Destructeur.
Definition: editorcommands.cpp:153
The CustomElementPart class This abstract class represents a primitive of the visual representation o...
Definition: customelementpart.h:40
void scale(const QRectF &before, const QRectF &after)
Definition: editorcommands.cpp:521
ScalePartsCommand(ElementScene *=nullptr, QUndoCommand *=nullptr)
Definition: editorcommands.cpp:444
ElementScene * m_scene
Element editor/view/scene the command should take place on.
Definition: editorcommands.h:57
~ChangeNamesCommand() override
Destructeur.
Definition: editorcommands.cpp:265
~ElementEditionCommand() override
Definition: editorcommands.cpp:59
void redo() override
Refait l'ajout.
Definition: editorcommands.cpp:228
The ElementScene class This class is the canvas allowing the visual edition of an electrial element....
Definition: elementscene.h:40
void undo() override
Annule le changement.
Definition: editorcommands.cpp:269
void applySendBackward(const QList< QGraphicsItem * > &)
Definition: editorcommands.cpp:397
DiagramContext m_elmt_kindInfo
element type
Definition: elementscene.h:70
NamesList names_after
List of new names.
Definition: editorcommands.h:167
QRectF new_rect_
new rect items should fit in
Definition: editorcommands.h:260
void setElementScene(ElementScene *)
Definition: editorcommands.cpp:72
QIcon tr
Definition: qeticons.cpp:206
void undo() override
Annule les changements de zValue.
Definition: editorcommands.cpp:319
void setTransformation(const QRectF &, const QRectF &)
ScalePartsCommand::setTransformation Define the transformation applied by this command.
Definition: editorcommands.cpp:503
void applyLower(const QList< QGraphicsItem * > &)
Definition: editorcommands.cpp:373
Definition: diagramcontext.h:56
QPointF movement
applied movement
Definition: editorcommands.h:117
void redo() override
Refait les changements de zValue.
Definition: editorcommands.cpp:324
void setElementInfo(const DiagramContext &dc)
ElementScene::setElementInfo.
Definition: elementscene.cpp:691
Definition: editorcommands.h:231
Definition: editorcommands.h:125
void redo() override
Refait le deplacement.
Definition: editorcommands.cpp:187