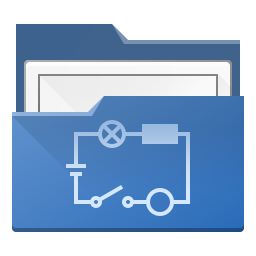 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef FILEELEMENTCOLLECTIONITEM2_H
19 #define FILEELEMENTCOLLECTIONITEM2_H
34 enum {
Type = UserType+2 };
39 bool hide_element =
false);
43 bool isDir()
const override;
47 QString
name()
const override;
60 bool hide_element =
false);
61 void populate(
bool set_data =
true,
bool hide_element =
false);
67 #endif // FILEELEMENTCOLLECTIONITEM2_H
bool setRootPath(const QString &path, bool set_data=true, bool hide_element=false)
FileElementCollectionItem::setRootPath Set path has root path for this file item. Use this function o...
Definition: fileelementcollectionitem.cpp:42
void populate(bool set_data=true, bool hide_element=false)
FileElementCollectionItem::populate Create the childs of this item.
Definition: fileelementcollectionitem.cpp:350
QList< QString > keys(KeyOrder=None) const
Definition: diagramcontext.cpp:49
void setUpIcon() override
FileElementCollectionItem::setUpIcon SetUp the icon of this item. Because icon use several memory,...
Definition: fileelementcollectionitem.cpp:301
QIcon nl
Definition: qeticons.cpp:203
FileElementCollectionItem()
FileElementCollectionItem::FileElementCollectionItem Constructor.
Definition: fileelementcollectionitem.cpp:30
virtual QString collectionPath() const =0
bool isDir() const override
FileElementCollectionItem::isDir.
Definition: fileelementcollectionitem.cpp:93
virtual bool isCollectionRoot() const =0
@ Type
Definition: fileelementcollectionitem.h:34
The ElementCollectionItem class This class represent a item (a directory or an element) in a element ...
Definition: elementcollectionitem.h:30
QString name() const override
FileElementCollectionItem::name.
Definition: fileelementcollectionitem.cpp:179
int type() const override
Definition: fileelementcollectionitem.h:35
void setPathName(const QString &path_name, bool set_data=true, bool hide_element=false)
FileElementCollectionItem::setPathName Set the name of this item in the file system path....
Definition: fileelementcollectionitem.cpp:333
QIcon icon() const
ElementLocation::icon.
Definition: elementslocation.cpp:756
QString fileSystemPath() const
FileElementCollectionItem::fileSystemPath.
Definition: fileelementcollectionitem.cpp:61
int rowForInsertItem(const QString &name)
ElementCollectionItem::rowForInsertItem Return the row for insert a new child item to this item with ...
Definition: elementcollectionitem.cpp:116
The FileElementCollectionItem class This class specialise ElementCollectionItem for manage a collecti...
Definition: fileelementcollectionitem.h:30
QIcon Folder
Definition: qeticons.cpp:94
static QString customElementsDirN()
QETApp::customElementsDirN like QString QETApp::customElementsDir but without "/" at the end.
Definition: qetapp.cpp:705
QVariant value(const QString &key) const
Definition: diagramcontext.cpp:100
QString dirPath() const
FileElementCollectionItem::dirPath.
Definition: fileelementcollectionitem.cpp:79
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
bool isCollectionRoot() const override
FileElementCollectionItem::isCollectionRoot.
Definition: fileelementcollectionitem.cpp:216
static QString commonElementsDirN()
QETApp::commonElementsDirN like QString QETApp::commonElementsDir but without "/" at the end.
Definition: qetapp.cpp:693
void setUpData() override
FileElementCollectionItem::setUpData SetUp the data of this item.
Definition: fileelementcollectionitem.cpp:264
Definition: nameslist.h:30
QString collectionPath() const override
FileElementCollectionItem::collectionPath.
Definition: fileelementcollectionitem.cpp:191
QString name() const
ElementLocation::name.
Definition: elementslocation.cpp:776
bool isCustomCollection() const
FileElementCollectionItem::isCustomCollection.
Definition: fileelementcollectionitem.cpp:238
DiagramContext elementInformations() const
ElementsLocation::elementInformations.
Definition: elementslocation.cpp:811
bool isElement() const override
FileElementCollectionItem::isElement.
Definition: fileelementcollectionitem.cpp:106
QIcon tr
Definition: qeticons.cpp:206
void addChildAtPath(const QString &collection_name) override
FileElementCollectionItem::addChildAtPath Ask to this item item to add a child with collection name c...
Definition: fileelementcollectionitem.cpp:249
bool isCommonCollection() const
FileElementCollectionItem::isCommonCollection.
Definition: fileelementcollectionitem.cpp:229
QString localName() override
FileElementCollectionItem::localName.
Definition: fileelementcollectionitem.cpp:115
Definition: diagramcontext.h:56
QString m_path
Definition: fileelementcollectionitem.h:64