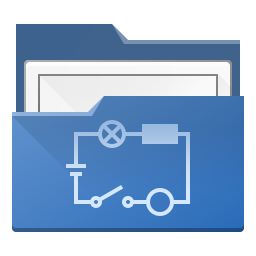 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
54 bool setParts(QList <CustomElementPart *> parts)
override;
Definition: lineeditor.h:32
void enableAnimation(bool animate=true)
QPropertyUndoCommand::enableAnimation True to enable animation.
Definition: qpropertyundocommand.cpp:92
void disconnectChangeConnections()
Definition: lineeditor.cpp:104
QList< CustomElementPart * > currentParts() const override
Definition: styleeditor.cpp:543
QIcon EndLineSimple
Definition: qeticons.cpp:91
LineEditor(QETElementEditor *, PartLine *=nullptr, QWidget *=nullptr)
Definition: lineeditor.cpp:32
bool setParts(QList< CustomElementPart * > parts) override
Definition: lineeditor.cpp:145
QUndoStack & undoStack()
ElementScene::undoStack.
Definition: elementscene.cpp:603
void updateLineEndLength1()
Met a jour la longueur de la premiere extremite.
Definition: lineeditor.cpp:204
QIcon EndLineCircle
Definition: qeticons.cpp:88
@ None
Regular line.
Definition: qet.h:194
void lineEditingFinishedY1()
Definition: lineeditor.cpp:293
QComboBox * end1_type
Definition: lineeditor.h:46
@ Simple
Base-less triangle.
Definition: qet.h:195
bool setPart(CustomElementPart *) override
LineEditor::setPart Specifie to this editor the part to edit. Note that an editor can accept or refus...
Definition: lineeditor.cpp:119
@ Diamond
Diamond.
Definition: qet.h:198
CustomElementPart * currentPart() const override
Definition: lineeditor.cpp:158
void updateLineEndLength2()
Met a jour la longueur de la seconde extremite.
Definition: lineeditor.cpp:247
QDoubleSpinBox * y2
Definition: lineeditor.h:45
void lineEditingFinishedX1()
Definition: lineeditor.cpp:268
StyleEditor * style_
Definition: lineeditor.h:44
void secondEndTypeChanged()
QDoubleSpinBox * x2
Definition: lineeditor.h:45
QPointF editedP2() const
LineEditor::editedP2.
Definition: lineeditor.cpp:178
QIcon EndLineNone
Definition: qeticons.cpp:90
Definition: styleeditor.h:35
void lineEditingFinishedY2()
Definition: lineeditor.cpp:343
Definition: elementitemeditor.h:34
void firstEndLengthChanged()
PartLine * part
Definition: lineeditor.h:43
QDoubleSpinBox * x1
Definition: lineeditor.h:45
void updateLineEndType2()
Met a jour le type de la seconde extremite.
Definition: lineeditor.cpp:226
virtual ElementScene * elementScene() const
Definition: elementitemeditor.cpp:39
void updateLineEndType1()
Met a jour le type de la premiere extremite.
Definition: lineeditor.cpp:183
QDoubleSpinBox * y1
Definition: lineeditor.h:45
void lineEditingFinishedX2()
Definition: lineeditor.cpp:318
~LineEditor() override
Destructeur.
Definition: lineeditor.cpp:92
QPointF editedP1() const
LineEditor::editedP1.
Definition: lineeditor.cpp:170
void firstEndTypeChanged()
Definition: partline.h:37
QDoubleSpinBox * end2_length
Definition: lineeditor.h:47
QList< CustomElementPart * > currentParts() const override
Definition: lineeditor.cpp:162
void activeConnections(bool)
LineEditor::activeConnections Enable/disable connection between editor widget and slot editingFinishe...
Definition: lineeditor.cpp:395
LineEditor(const LineEditor &)
QDoubleSpinBox * end1_length
Definition: lineeditor.h:47
bool setParts(QList< CustomElementPart * >) override
StyleEditor::setParts Set several parts to edit by this editor. Note : editor can accept or refuse to...
Definition: styleeditor.cpp:510
QComboBox * end2_type
Definition: lineeditor.h:46
QIcon EndLineTriangle
Definition: qeticons.cpp:92
@ Circle
Circle.
Definition: qet.h:197
The CustomElementPart class This abstract class represents a primitive of the visual representation o...
Definition: customelementpart.h:40
@ Triangle
Triangle.
Definition: qet.h:196
QIcon EndLineDiamond
Definition: qeticons.cpp:89
void secondEndLengthChanged()
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
bool m_locked
Definition: lineeditor.h:48
QIcon tr
Definition: qeticons.cpp:206
void setUpChangeConnections()
setUpChangeConnections Setup the connection from the line(s) to the widget, to update it when the lin...
Definition: lineeditor.cpp:95
QList< QMetaObject::Connection > m_change_connections
Definition: lineeditor.h:49
Definition: qetelementeditor.h:33
QVariant property(const char *name) const override
Definition: customelementgraphicpart.h:297
void updateForm() override
LineEditor::updateForm Update the value of the widgets.
Definition: lineeditor.cpp:372