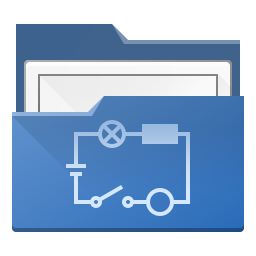 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_SLASH_DIMENSION_WIDGET_H
19 #define TITLEBLOCK_SLASH_DIMENSION_WIDGET_H
40 QLabel *
label()
const;
@ RelativeToTotalLength
the length is just a fraction of the total available length
Definition: qet.h:147
int value
Numeric value.
Definition: dimension.h:34
QET::TitleBlockColumnLength type
Kind of length.
Definition: dimension.h:33
TitleBlockColumnLength
enum used to specify the type of a length
Definition: qet.h:145
Definition: dimension.h:26
@ Absolute
the length is absolute and should be applied as is
Definition: qet.h:146
QIcon tr
Definition: qeticons.cpp:206
QIcon Cancel
Definition: qeticons.cpp:34
@ RelativeToRemainingLength
the length is just a fraction of the length that is still available when other types of lengths have ...
Definition: qet.h:148