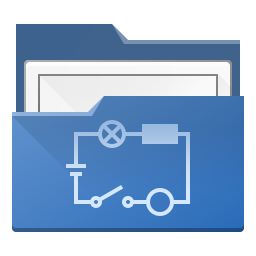 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTS_LOCATION_H
19 #define ELEMENTS_LOCATION_H
23 #include "pugixml.hpp"
86 QDomElement
xml()
const;
87 pugi::xml_document
pugiXml()
const;
88 bool setXml(
const QDomDocument &xml_document)
const;
static int MetaTypeId
Id of the corresponding Qt meta type.
Definition: elementslocation.h:104
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
bool fetchElement(ElementsLocation &location)
ElementsCollectionCache::fetchElement Retrieve the data for a given element, using the cache if avail...
Definition: elementscollectioncache.cpp:161
static ElementsCollectionCache * collectionCache()
QETApp::collectionCache.
Definition: qetapp.cpp:358
XmlElementCollection * embeddedElementCollection() const
QETProject::embeddedCollection.
Definition: qetproject.cpp:236
bool setXml(const QDomDocument &xml_document) const
ElementsLocation::setXml Replace the current xml description by xml_document; The document element of...
Definition: elementslocation.cpp:667
void fromXml(const QDomElement &, const QString &="property")
Definition: diagramcontext.cpp:157
bool isReadOnly() const
QETProject::isReadOnly.
Definition: qetproject.cpp:924
Q_DECLARE_METATYPE(NamesList)
QIcon nl
Definition: qeticons.cpp:203
QString path() const
ElementsLocation::path.
Definition: elementslocation.cpp:206
QString fileName() const
ElementLocation::fileName.
Definition: elementslocation.cpp:793
ElementsLocation()
ElementsLocation::ElementsLocation Constructor.
Definition: elementslocation.cpp:36
QString m_collection_path
Definition: elementslocation.h:96
Definition: elementscollectioncache.h:31
bool isWritable() const
ElementsLocation::isWritable.
Definition: elementslocation.cpp:499
The XmlElementCollection class This class represent a collection of elements stored to xml.
Definition: xmlelementcollection.h:34
QUuid uuid() const
ElementsLocation::uuid.
Definition: elementslocation.cpp:737
bool exist(const QString &path) const
XmlElementCollection::exist Return true if the path path exist in this collection.
Definition: xmlelementcollection.cpp:569
bool isFileSystem() const
ElementsLocation::isFileSystem.
Definition: elementslocation.cpp:428
void setProject(QETProject *)
ElementsLocation::setProject.
Definition: elementslocation.cpp:377
bool operator!=(const ElementsLocation &) const
ElementsLocation::operator != Operateur de comparaison.
Definition: elementslocation.cpp:127
bool writeXmlFile(const QDomDocument &xml_document, const QString &file_path, QString *error_message=nullptr)
QETXML::writeXmlFile Export an XML document to an UTF-8 text file indented with 4 spaces,...
Definition: qetxml.cpp:257
QDomElement element(const QString &path) const
XmlElementCollection::element.
Definition: xmlelementcollection.cpp:291
bool isElement() const
ElementsLocation::isElement.
Definition: elementslocation.cpp:412
QString baseName() const
ElementsLocation::baseName.
Definition: elementslocation.cpp:142
QIcon icon() const
ElementLocation::icon.
Definition: elementslocation.cpp:756
ElementsLocation parent() const
ElementsLocation::parent.
Definition: elementslocation.cpp:346
virtual ~ElementsLocation()
ElementsLocation::~ElementsLocation Destructeur.
Definition: elementslocation.cpp:56
bool isNull() const
ElementsLocation::isNull.
Definition: elementslocation.cpp:387
QDomElement xml() const
ElementsLocation::xml.
Definition: elementslocation.cpp:575
bool addToPath(const QString &)
ElementsLocation::addToPath Add a string to the actual path of this location.
Definition: elementslocation.cpp:320
bool isDirectory() const
ElementsLocation::isDirectory.
Definition: elementslocation.cpp:420
bool addElementDefinition(const QString &dir_path, const QString &elmt_name, const QDomElement &xml_definition)
XmlElementCollection::addElementDefinition Add the élément defintion xml_definition in the directory ...
Definition: xmlelementcollection.cpp:477
QString projectCollectionPath() const
ElementsLocation::projectCollectionPath.
Definition: elementslocation.cpp:176
pugi::xml_document pugiXml() const
ElementsLocation::pugiXml.
Definition: elementslocation.cpp:610
static int projectId(const QETProject *)
QETApp::projectId.
Definition: qetapp.cpp:2433
QString fileSystemPath() const
ElementsLocation::fileSystemPath.
Definition: elementslocation.cpp:193
QString m_file_system_path
Definition: elementslocation.h:97
static QString customElementsDirN()
QETApp::customElementsDirN like QString QETApp::customElementsDir but without "/" at the end.
Definition: qetapp.cpp:705
bool isProject() const
ElementsLocation::isProject.
Definition: elementslocation.cpp:459
QDebug operator<<(QDebug debug, const ElementsLocation &location)
operator << debug for processing ElementsLocation
Definition: elementslocation.cpp:830
QDomElement directory(const QString &path) const
XmlElementCollection::directory.
Definition: xmlelementcollection.cpp:309
static ElementPictureFactory * instance()
instance
Definition: elementpicturefactory.h:55
ElementsLocation & operator=(const ElementsLocation &)
ElementsLocation::operator = Assignment operator.
Definition: elementslocation.cpp:96
QString collectionPath(bool protocol=true) const
ElementsLocation::collectionPath Return the path of the represented element relative to collection if...
Definition: elementslocation.cpp:160
NamesList nameList()
ElementsLocation::nameList.
Definition: elementslocation.cpp:532
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
bool isCommonCollection() const
ElementsLocation::isCommonCollection.
Definition: elementslocation.cpp:440
std::stringstream m_string_stream
Definition: elementslocation.h:100
Definition: qetproject.h:51
static QString commonElementsDirN()
QETApp::commonElementsDirN like QString QETApp::commonElementsDir but without "/" at the end.
Definition: qetapp.cpp:693
Definition: nameslist.h:30
QETProject * m_project
Definition: elementslocation.h:98
QString name() const
ElementLocation::name.
Definition: elementslocation.cpp:776
static QETProject * project(const uint &)
QETApp::project.
Definition: qetapp.cpp:2416
XmlElementCollection * projectCollection() const
ElementsLocation::projectCollection.
Definition: elementslocation.cpp:519
QPixmap pixmap() const
Definition: elementscollectioncache.cpp:200
DiagramContext elementInformations() const
ElementsLocation::elementInformations.
Definition: elementslocation.cpp:811
QString toString() const
ElementsLocation::toString.
Definition: elementslocation.cpp:396
QDebug operator<<(QDebug debug, const ElementsLocation &location)
operator << debug for processing ElementsLocation
Definition: elementslocation.cpp:830
QETProject * project() const
ElementsLocation::project.
Definition: elementslocation.cpp:365
void setPath(const QString &path)
ElementsLocation::setPath Set the path of this item. The path can be relative to a collection (start ...
Definition: elementslocation.cpp:217
bool isCustomCollection() const
ElementsLocation::isCustomCollection.
Definition: elementslocation.cpp:450
Definition: diagramcontext.h:56
bool operator==(const ElementsLocation &) const
ElementsLocation::operator ==.
Definition: elementslocation.cpp:113