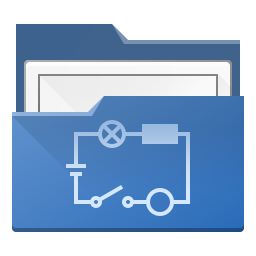 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef PART_POLYGON_H
19 #define PART_POLYGON_H
54 enum {
Type = UserType + 1105 };
60 void paint(QPainter *,
const QStyleOptionGraphicsItem *, QWidget *)
override;
62 QString
name()
const override {
return(
QObject::tr(
"polygone",
"element part name")); }
63 QString
xmlName()
const override {
return(QString(
"polygon")); }
64 void fromXml(
const QDomElement &)
override;
65 const QDomElement
toXml(QDomDocument &)
const override;
67 QPainterPath
shape ()
const override;
80 void addPoint (
const QPointF &point);
91 QVariant
itemChange(GraphicsItemChange change, const QVariant &value) override;
QList< QPointF > saved_points_
Definition: partpolygon.h:109
void paint(QPainter *, const QStyleOptionGraphicsItem *, QWidget *) override
PartPolygon::paint Draw this polygon.
Definition: partpolygon.cpp:62
QAction * m_remove_point
Definition: partpolygon.h:115
QPointF snapToGrid(QPointF point)
ElementScene::snapToGrid Rounds the coordinates of the point passed as a parameter so that this point...
Definition: elementscene.cpp:1270
@ Type
Definition: qetgraphicshandleritem.h:42
QUndoStack & undoStack()
ElementScene::undoStack.
Definition: elementscene.cpp:603
void handlerMousePressEvent(QetGraphicsHandlerItem *qghi, QGraphicsSceneMouseEvent *event)
PartPolygon::handlerMousePressEvent.
Definition: partpolygon.cpp:417
bool m_closed
Definition: partpolygon.h:108
The CustomElementGraphicPart class This class is the base for all home-made primitive like line,...
Definition: customelementgraphicpart.h:36
void removeHandler()
PartPolygon::removeHandler Remove the handlers of this item.
Definition: partpolygon.cpp:498
QVector< QetGraphicsHandlerItem * > m_handler_vector
Definition: partpolygon.h:113
@ Type
Definition: partpolygon.h:54
void setHandlerColor(QPointF pos, const QColor &color) final
PartPolygon::setHandlerColor Set the handler at pos pos (in polygon coordinate) to color color.
Definition: partpolygon.cpp:266
void fromXml(const QDomElement &) override
PartPolygon::fromXml Import the properties of this polygon from a xml element.
Definition: partpolygon.cpp:85
void stylesToXml(QDomElement &) const
CustomElementGraphicPart::stylesToXml Write the curent style to xml element. The style are stored lik...
Definition: customelementgraphicpart.cpp:162
QString xmlName() const override
Definition: partpolygon.h:63
QPainterPath shadowShape() const override
Definition: partpolygon.cpp:577
void setNewValue(const QVariant &new_value)
QPropertyUndoCommand::setNewValue Set the new value of the property (set with redo) to new_value.
Definition: qpropertyundocommand.cpp:83
bool isClosed() const
Definition: partpolygon.h:84
QPolygonF m_polygon
Definition: partpolygon.h:110
QPolygonF polygon
Definition: partpolygon.h:38
void removeLastPoint()
PartPolygon::removeLastPoint Remove the last point of polygon.
Definition: partpolygon.cpp:243
void setPolygon(const QPolygonF &polygon)
PartPolygon::setPolygon Sets the item's polygon to be the given polygon.
Definition: partpolygon.cpp:205
QAction * m_insert_point
Definition: partpolygon.h:114
void adjusteHandlerPos()
PartPolygon::adjusteHandlerPos.
Definition: partpolygon.cpp:393
void addPoint(const QPointF &point)
PartPolygon::addPoint Add new point to polygon.
Definition: partpolygon.cpp:219
The PartPolygon class This class represents a polygon primitive which may be used to compose the draw...
Definition: partpolygon.h:34
~PartPolygon() override
PartPolygon::~PartPolygon.
Definition: partpolygon.cpp:49
void handleUserTransformation(const QRectF &, const QRectF &) override
PartPolygon::handleUserTransformation Handle the user-induced transformation from initial_selection_r...
Definition: partpolygon.cpp:175
void stylesFromXml(const QDomElement &)
CustomElementGraphicPart::stylesFromXml Read the style used by this, from a xml element.
Definition: customelementgraphicpart.cpp:508
void setLastPoint(const QPointF &point)
PartPolygon::setLastPoint Set the last point of polygon to point.
Definition: partpolygon.cpp:230
void addHandler()
PartPolygon::addHandler Add handlers for this item.
Definition: partpolygon.cpp:478
void resetAllHandlerColor() final
PartPolygon::resetAllHandlerColor Reset the color of every handlers.
Definition: partpolygon.cpp:279
QPainterPath shape() const override
PartPolygon::shape.
Definition: partpolygon.cpp:562
QETElementEditor * editor() const
ElementScene::editor.
Definition: elementscene.cpp:683
QPointF m_context_menu_pos
Definition: partpolygon.h:116
QRectF sceneGeometricRect() const override
PartPolygon::sceneGeometricRect.
Definition: partpolygon.cpp:153
bool sceneEventFilter(QGraphicsItem *watched, QEvent *event) override
PartPolygon::sceneEventFilter.
Definition: partpolygon.cpp:332
PartPolygon(const PartPolygon &)
void handlerMouseMoveEvent(QetGraphicsHandlerItem *qghi, QGraphicsSceneMouseEvent *event)
PartPolygon::handlerMouseMoveEvent.
Definition: partpolygon.cpp:431
QET::ScalingMethod preferredScalingMethod() const override
PartPolygon::preferredScalingMethod This method is called by the decorator when it needs to determine...
Definition: partpolygon.cpp:188
QRectF boundingRect() const override
PartPolygon::boundingRect.
Definition: partpolygon.cpp:595
The QetGraphicsHandlerItem class This graphics item represents a point, destined to be used as an han...
Definition: qetgraphicshandleritem.h:37
int type() const override
Definition: partpolygon.h:59
bool m_hovered
Definition: customelementgraphicpart.h:323
void startUserTransformation(const QRectF &) override
PartPolygon::startUserTransformation Start the user-induced transformation, provided this primitive i...
Definition: partpolygon.cpp:163
QPropertyUndoCommand * m_undo_command
Definition: partpolygon.h:111
const QDomElement toXml(QDomDocument &) const override
PartPolygon::toXml Export this polygin in xml.
Definition: partpolygon.cpp:116
void insertPoint()
PartPolygon::insertPoint Insert a point in this polygone.
Definition: partpolygon.cpp:511
void contextMenuEvent(QGraphicsSceneContextMenuEvent *event) override
Definition: partpolygon.cpp:366
#define SHADOWS_HEIGHT
Definition: customelementgraphicpart.h:37
Color color
Definition: customelementgraphicpart.h:44
void removePoint()
PartPolygon::removePoint remove a point on this polygon.
Definition: partpolygon.cpp:528
virtual ElementScene * elementScene() const
Definition: customelementpart.cpp:37
bool closed
Definition: partpolygon.h:37
QList< QPointF > mapPoints(const QRectF &, const QRectF &, const QList< QPointF > &)
Definition: customelementpart.cpp:69
void sceneSelectionChanged()
PartPolygon::sceneSelectionChanged When the scene selection change, if there are several primitive se...
Definition: partpolygon.cpp:466
ScalingMethod
Supported types of interactive scaling, typically for a single element primitive.
Definition: qet.h:81
void applyStylesToQPainter(QPainter &) const
CustomElementGraphicPart::applyStylesToQPainter Apply the current style to the QPainter.
Definition: customelementgraphicpart.cpp:885
int m_vector_index
Definition: partpolygon.h:112
qreal penWeight() const
CustomElementGraphicPart::penWeight.
Definition: customelementgraphicpart.cpp:109
@ RoundScaleRatios
adjust the scaling movement so that the induced scaling ratios are rounded
Definition: qet.h:84
static QPolygonF polygonForInsertPoint(const QPolygonF &old_polygon, bool closed, const QPointF &pos)
QetGraphicsHandlerUtility::polygonForInsertPoint.
Definition: qetgraphicshandlerutility.cpp:213
void contextMenu(QPoint p, QList< QAction * > actions=QList< QAction * >())
QETElementEditor::contextMenu Display a context menu, with all available action.
Definition: qetelementeditor.cpp:431
@ Normal
Definition: elementscene.h:46
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
void handlerMouseReleaseEvent(QetGraphicsHandlerItem *qghi, QGraphicsSceneMouseEvent *event)
PartPolygon::handlerMouseReleaseEvent.
Definition: partpolygon.cpp:451
static QVector< QetGraphicsHandlerItem * > handlerForPoint(const QVector< QPointF > &points, int size=10)
QetGraphicsHandlerItem::handlerForPoint.
Definition: qetgraphicshandleritem.cpp:87
QIcon Add
Definition: qeticons.cpp:24
QIcon tr
Definition: qeticons.cpp:206
QVariant itemChange(GraphicsItemChange change, const QVariant &value) override
PartPolygon::itemChange.
Definition: partpolygon.cpp:292
PartPolygon(QETElementEditor *editor, QGraphicsItem *parent=nullptr)
PartPolygon::PartPolygon Constructor.
Definition: partpolygon.cpp:33
bool attributeIsAReal(const QDomElement &, const QString &, qreal *=nullptr)
Definition: qet.cpp:231
Definition: qetelementeditor.h:33
void setClosed(bool close)
Definition: partpolygon.cpp:252
bool isUseless() const override
PartPolygon::isUseless.
Definition: partpolygon.cpp:136
void drawShadowShape(QPainter *painter)
CustomElementGraphicPart::drawShadowShape Draw a transparent blue shadow arround the shape of this it...
Definition: customelementgraphicpart.cpp:1240
QString name() const override
Definition: partpolygon.h:62
QIcon Remove
Definition: qeticons.cpp:155