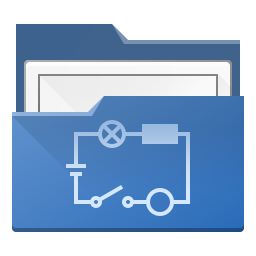 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_SLASH_TEMPLATE_CELL_WIDGET_H
19 #define TITLEBLOCK_SLASH_TEMPLATE_CELL_WIDGET_H
92 const QString &)
const;
94 const QVariant &)
const;
void setHelpText(const QString &text)
Definition: namelistdialog.cpp:55
void setInformationText(const QString &text)
Definition: namelistdialog.cpp:42
@ TextCell
Definition: titleblockcell.h:30
static QFont fontForCell(const TitleBlockCell &)
TitleBlockTemplate::fontForCell.
Definition: titleblocktemplate.cpp:80
The NameListDialog class Provide a dialog for let user define localized string;.
Definition: namelistdialog.h:34
The TitleBlockTemplate class This class represents an title block template for an electric diagram....
Definition: titleblocktemplate.h:36
Definition: templatecommands.h:33
@ EmptyCell
Definition: titleblockcell.h:29
@ LogoCell
Definition: titleblockcell.h:31
Definition: nameslist.h:30
QIcon InsertImage
Definition: qeticons.cpp:116
static QString attributeName(const QString &)
Definition: titleblockcell.cpp:108
QIcon tr
Definition: qeticons.cpp:206
Definition: titleblockcell.h:26
NameListWidget * namelistWidget() const
NameListDialog::namelistWidget.
Definition: namelistdialog.cpp:51