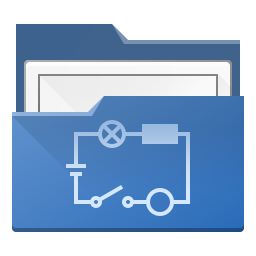 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_SLASH_TEMPLATE_LOGO_MANAGER
19 #define TITLEBLOCK_SLASH_TEMPLATE_LOGO_MANAGER
Definition: templatelogomanager.h:27
QLineEdit * logo_name_
current logo name
Definition: templatelogomanager.h:71
~TitleBlockTemplateLogoManager() override
Definition: templatelogomanager.cpp:40
QHBoxLayout * hlayout1_
horizontal layouts
Definition: templatelogomanager.h:63
QLabel * logo_name_label_
"name:" label
Definition: templatelogomanager.h:70
QString currentLogo() const
Definition: templatelogomanager.cpp:47
void renameLogo()
Definition: templatelogomanager.cpp:332
QLabel * logos_label_
simple displayed label
Definition: templatelogomanager.h:64
QLabel * logo_type_
current logo type
Definition: templatelogomanager.h:73
void initWidgets()
Definition: templatelogomanager.cpp:74
void logosChanged(const TitleBlockTemplate *)
QPushButton * delete_button_
button to delete an embedded logo
Definition: templatelogomanager.h:68
void fillView()
Definition: templatelogomanager.cpp:136
void addLogo()
Definition: templatelogomanager.cpp:264
QVBoxLayout * vlayout0_
Definition: templatelogomanager.h:62
QDialogButtonBox * buttons_
ok/cancel buttons
Definition: templatelogomanager.h:74
void emitLogosChangedSignal()
Definition: templatelogomanager.cpp:67
QIcon DocumentExport
Definition: qeticons.cpp:50
bool isReadOnly() const
Definition: templatelogomanager.cpp:60
QSize iconsize() const
Definition: templatelogomanager.cpp:170
void updateLogoInformations(QListWidgetItem *, QListWidgetItem *)
Definition: templatelogomanager.cpp:245
The TitleBlockTemplate class This class represents an title block template for an electric diagram....
Definition: titleblocktemplate.h:36
QString confirmLogoName(const QString &)
Definition: templatelogomanager.cpp:182
QListWidget * logos_view_
area showing the logos
Definition: templatelogomanager.h:65
QHBoxLayout * hlayout0_
Definition: templatelogomanager.h:63
QVBoxLayout * vlayout1_
vertical layouts
Definition: templatelogomanager.h:62
QPushButton * add_button_
button to add a new logo
Definition: templatelogomanager.h:66
bool read_only_
Whether this logo manager should allow logo edition (renaming, addition, deletion)
Definition: templatelogomanager.h:76
QIcon EditRename
Definition: qeticons.cpp:69
void setReadOnly(bool)
Definition: templatelogomanager.cpp:375
void removeLogo()
Definition: templatelogomanager.cpp:319
void exportLogo()
Definition: templatelogomanager.cpp:296
QGroupBox * logo_box_
current logo properties box
Definition: templatelogomanager.h:69
QIcon InsertImage
Definition: qeticons.cpp:116
QPushButton * export_button_
button to export an embedded logo
Definition: templatelogomanager.h:67
QMessageBox::StandardButton warning(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:68
QDir open_dialog_dir_
last opened directory
Definition: templatelogomanager.h:75
TitleBlockTemplate * managed_template_
title block template which this class manages logos
Definition: templatelogomanager.h:61
QMessageBox::StandardButton critical(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:23
QIcon Add
Definition: qeticons.cpp:24
QIcon tr
Definition: qeticons.cpp:206
TitleBlockTemplateLogoManager(TitleBlockTemplate *, QWidget *=nullptr)
Definition: templatelogomanager.cpp:29
QIcon Cancel
Definition: qeticons.cpp:34
QPushButton * rename_button_
button to rename the current logo
Definition: templatelogomanager.h:72
QIcon Remove
Definition: qeticons.cpp:155