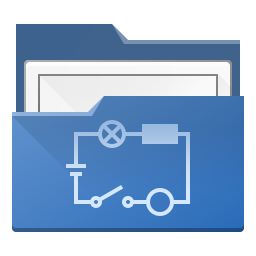 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTPICTUREFACTORY_H
19 #define ELEMENTPICTUREFACTORY_H
22 #include <QSharedPointer>
30 class QGraphicsSimpleTextItem;
95 bool build(
const ElementsLocation &location, QPicture *picture=
nullptr, QPicture *low_picture=
nullptr);
113 #endif // ELEMENTPICTUREFACTORY_H
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
QHash< QUuid, QPicture > m_pictures_H
Definition: elementpicturefactory.h:106
void parsePolygon(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:450
QList< QLineF > m_lines
Definition: elementpicturefactory.h:42
~ElementPictureFactory()
Definition: elementpicturefactory.cpp:129
void parseCircle(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:406
const QString version
QElectroTech version (as string, used to mark projects and elements XML documents)
Definition: qet.h:32
static ElementPictureFactory * m_factory
Definition: elementpicturefactory.h:110
static uint requiredLengthForEndType(const Qet::EndType &)
PartLine::requiredLengthForEndType.
Definition: partline.cpp:54
QHash< QUuid, QPicture > m_low_pictures_H
Definition: elementpicturefactory.h:107
void setPainterStyle(const QDomElement &dom, QPainter &painter) const
ElementPictureFactory::setPainterStyle apply the style store in dom to painter.
Definition: elementpicturefactory.cpp:555
@ Simple
Base-less triangle.
Definition: qet.h:195
@ Diamond
Diamond.
Definition: qet.h:198
QUuid uuid() const
ElementsLocation::uuid.
Definition: elementslocation.cpp:737
ElementPictureFactory operator=(const ElementPictureFactory &)
QList< QRectF > m_circles
Definition: elementpicturefactory.h:44
bool build(const ElementsLocation &location, QPicture *picture=nullptr, QPicture *low_picture=nullptr)
ElementPictureFactory::build Build the picture from location.
Definition: elementpicturefactory.cpp:147
Definition: elementpicturefactory.h:41
static void dropInstance()
dropInstance Drop the instance of factory
Definition: elementpicturefactory.h:73
void getPictures(const ElementsLocation &location, QPicture &picture, QPicture &low_picture)
ElementPictureFactory::getPictures Set the picture of the element at location. Note,...
Definition: elementpicturefactory.cpp:42
void parseText(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:487
QPixmap pixmap(const ElementsLocation &location)
ElementPictureFactory::pixmap.
Definition: elementpicturefactory.cpp:76
ElementPictureFactory::primitives getPrimitives(const ElementsLocation &location)
ElementPictureFactory::getPrimitives.
Definition: elementpicturefactory.cpp:120
QDomElement xml() const
ElementsLocation::xml.
Definition: elementslocation.cpp:575
QList< QGraphicsSimpleTextItem * > m_texts
Definition: elementpicturefactory.h:47
QList< QRectF > m_rectangles
Definition: elementpicturefactory.h:43
QList< QVector< QPointF > > m_polygons
Definition: elementpicturefactory.h:45
ElementPictureFactory()
Definition: elementpicturefactory.h:90
QHash< QUuid, primitives > m_primitives_H
Definition: elementpicturefactory.h:109
EndType
This enum lists the various available endings for line primitives when drawing an electrical element.
Definition: qet.h:193
pugi::xml_document pugiXml() const
ElementsLocation::pugiXml.
Definition: elementslocation.cpp:610
void parseLine(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:259
QList< QVector< qreal > > m_arcs
Definition: elementpicturefactory.h:46
The ElementPictureFactory class This class is singleton factory, use to create and get the picture us...
Definition: elementpicturefactory.h:38
static ElementPictureFactory * instance()
instance
Definition: elementpicturefactory.h:55
static QList< QPointF > fourEndPoints(const QPointF &, const QPointF &, const qreal &)
PartLine::fourEndPoints Return the four interesting point needed to draw the shape at extremity of li...
Definition: partline.cpp:601
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
static Qet::EndType endTypeFromString(const QString &)
Definition: qet.cpp:506
@ Circle
Circle.
Definition: qet.h:197
QHash< QUuid, QPixmap > m_pixmap_H
Definition: elementpicturefactory.h:108
ElementPictureFactory(const ElementPictureFactory &)
void parseEllipse(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:382
static QFont diagramTextsFont(qreal=-1.0)
QETApp::diagramTextsFont The font to use By default the font is "sans Serif" and size 9.
Definition: qetapp.cpp:1111
bool attributeIsAnInteger(const QDomElement &, const QString &, int *=nullptr)
Definition: qet.cpp:210
void parseArc(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:423
void parseElement(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:248
@ Triangle
Triangle.
Definition: qet.h:196
void parseRect(const QDomElement &dom, QPainter &painter, primitives &prim) const
Definition: elementpicturefactory.cpp:358
QIcon tr
Definition: qeticons.cpp:206
bool attributeIsAReal(const QDomElement &, const QString &, qreal *=nullptr)
Definition: qet.cpp:231