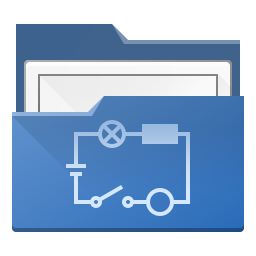 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTINFOWIDGET_H
19 #define ELEMENTINFOWIDGET_H
48 void apply()
override;
50 QString
title()
const override {
return tr(
"Informations");}
71 Ui::ElementInfoWidget *
ui;
76 #endif // ELEMENTINFOWIDGET_H
void remove(const QString &key)
DiagramContext::remove.
Definition: diagramcontext.cpp:42
static QStringList elementInfoKeys()
QETApp::elementInfoKeys.
Definition: qetapp.cpp:366
void elementInfoChange(DiagramContext old_info, DiagramContext new_info)
static QString elementTranslatedInfoKey(const QString &)
ElementsProperties::translatedInfo Return the translated information key given by info If info don't ...
Definition: qetapp.cpp:400
Definition: autonumberingdockwidget.h:25
bool addValue(const QString &, const QVariant &, bool show=true)
Definition: diagramcontext.cpp:91
QIcon tr
Definition: qeticons.cpp:206
Definition: diagramcontext.h:56