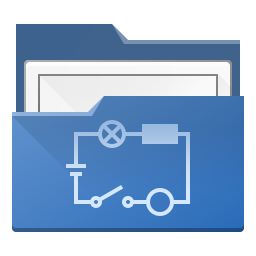 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
31 static void dxfBegin (
const QString&);
32 static void dxfEnd(
const QString&);
39 static void drawArc(
const QString&,
46 static void drawDonut(QString,
double,
double,
double,
int);
58 const int &colorcode);
62 const int &colorcode);
69 const int &colorcode);
72 const int &colorcode);
74 static void drawLine(
const QString &filapath,
80 static void drawLine(
const QString &filepath,
82 const int &colorcode);
102 bool leftAlign =
false,
117 #endif // CREATEDXF_H
static const double sheetHeight
Definition: createdxf.h:112
static double yScale
Definition: createdxf.h:114
Definition: createdxf.h:27
static void dxfBegin(const QString &)
Definition: createdxf.cpp:42
static void dxfEnd(const QString &)
Definition: createdxf.cpp:232
static long RGBcodeTable[]
Definition: createdxf.h:109
static int getcolorCode(const long red, const long green, const long blue)
Createdxf::getcolorCode This function returns the ACI color which is the "nearest" color to the color...
Definition: createdxf.cpp:389
static void drawLine(const QString &filapath, double, double, double, double, const int &clorcode)
Definition: createdxf.cpp:290
static void drawCircle(const QString &, double, double, double, int)
Definition: createdxf.cpp:256
static void drawRectangle(const QString &filepath, double, double, double, double, const int &colorcode)
Definition: createdxf.cpp:565
static void drawText(const QString &, const QString &, double, double, double, double, int)
Createdxf::drawText draw simple text in dxf format without any alignment specified.
Definition: createdxf.cpp:736
static double xScale
Definition: createdxf.h:113
static void drawArc(const QString &, double x, double y, double rad, double startAngle, double endAngle, int color)
Createdxf::drawArc draw arc in dx format.
Definition: createdxf.cpp:683
static const double sheetWidth
Definition: createdxf.h:111
static void drawEllipse(const QString &filepath, const QRectF &rect, const int &colorcode)
Createdxf::drawEllipse Conveniance function for draw ellipse.
Definition: createdxf.cpp:556
static void drawArcEllipse(const QString &file_path, qreal x, qreal y, qreal w, qreal h, qreal startAngle, qreal spanAngle, qreal hotspot_x, qreal hotspot_y, qreal rotation_angle, const int &colorcode)
Definition: createdxf.cpp:426
static void drawTextAligned(const QString &fileName, const QString &text, double x, double y, double height, double rotation, double oblique, int hAlign, int vAlign, double xAlign, double xScale, int colour, bool leftAlign=false, float scale=0)
Definition: createdxf.cpp:781
Createdxf()
Definition: createdxf.cpp:32
~Createdxf()
Definition: createdxf.cpp:37
static void drawDonut(QString, double, double, double, int)
static QPointF rotation_transformed(qreal, qreal, qreal, qreal, qreal)
Definition: exportdialog.cpp:668