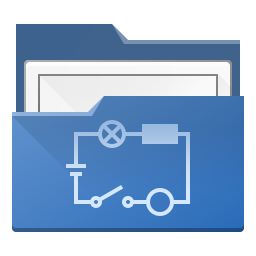 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef XREFPROPERTIES_H
19 #define XREFPROPERTIES_H
22 #include <QStringList>
44 const QString = QString())
const override;
46 const QString = QString())
override;
47 QDomElement
toXml (QDomDocument &xml_document)
const override;
48 bool fromXml(
const QDomElement &xml_element)
override;
93 #endif // XREFPROPERTIES_H
bool m_show_power_ctc
Definition: xrefproperties.h:81
void setSnapTo(const SnapTo st)
Definition: xrefproperties.h:61
QString m_slave_label
Definition: xrefproperties.h:88
QString m_key
Definition: xrefproperties.h:90
void fromSettings(const QSettings &settings, const QString=QString()) override
XRefProperties::fromSettings load from settings.
Definition: xrefproperties.cpp:73
Qt::AlignmentFlag getXrefPos() const
Definition: xrefproperties.h:65
bool fromXml(const QDomElement &xml_element) override
XRefProperties::fromXml Load from xml.
Definition: xrefproperties.cpp:133
void setMasterLabel(const QString master)
Definition: xrefproperties.h:69
@ Cross
Definition: xrefproperties.h:34
void setSlaveLabel(const QString slave)
Definition: xrefproperties.h:72
QDomElement toXml(QDomDocument &xml_document) const override
XRefProperties::toXml Save to xml.
Definition: xrefproperties.cpp:99
void setOffset(const int offset)
Definition: xrefproperties.h:75
@ Label
Definition: xrefproperties.h:40
Qt::AlignmentFlag m_xref_pos
Definition: xrefproperties.h:84
void setPrefix(const QString &key, const QString &value)
Definition: xrefproperties.h:66
int offset() const
Definition: xrefproperties.h:76
int m_offset
Definition: xrefproperties.h:89
bool operator==(const XRefProperties &xrp) const
Definition: xrefproperties.cpp:184
SnapTo
Definition: xrefproperties.h:38
The XRefProperties class this class store properties used by XrefItem.
Definition: xrefproperties.h:29
QString masterLabel() const
Definition: xrefproperties.h:70
QString m_master_label
Definition: xrefproperties.h:87
QString slaveLabel() const
Definition: xrefproperties.h:73
void setXrefPos(const Qt::AlignmentFlag xref)
Definition: xrefproperties.h:64
@ Contacts
Definition: xrefproperties.h:35
SnapTo m_snap_to
Definition: xrefproperties.h:83
void setShowPowerContac(const bool a)
Definition: xrefproperties.h:55
XRefProperties()
XRefProperties::XRefProperties Default Constructor.
Definition: xrefproperties.cpp:26
DisplayHas
Definition: xrefproperties.h:33
QStringList m_prefix_keys
Definition: xrefproperties.h:86
void setKey(QString &key)
Definition: xrefproperties.h:78
QHash< QString, QString > m_prefix
Definition: xrefproperties.h:85
bool showPowerContact() const
Definition: xrefproperties.h:56
DisplayHas m_display
Definition: xrefproperties.h:82
QString prefix(const QString &key) const
Definition: xrefproperties.h:67
@ Bottom
Definition: xrefproperties.h:39
void setDisplayHas(const DisplayHas dh)
Definition: xrefproperties.h:58
DisplayHas displayHas() const
Definition: xrefproperties.h:59
The PropertiesInterface class This class is an interface for have common way to use properties in QEl...
Definition: propertiesinterface.h:31
bool operator!=(const XRefProperties &xrp) const
Definition: xrefproperties.cpp:194
SnapTo snapTo() const
Definition: xrefproperties.h:62
void toSettings(QSettings &settings, const QString=QString()) const override
XRefProperties::toSettings Save to settings.
Definition: xrefproperties.cpp:44
static QHash< QString, XRefProperties > defaultProperties()
XRefProperties::defaultProperties.
Definition: xrefproperties.cpp:165