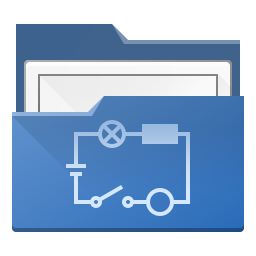 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
41 bool setParts(QList <CustomElementPart *>)
override;
63 #endif // TEXTEDITOR_H
void setUpChangeConnection(QPointer< PartText > part)
Definition: texteditor.cpp:72
QList< QMetaObject::Connection > m_change_connection
Definition: texteditor.h:60
Definition: texteditor.h:32
CustomElementPart * currentPart() const override
TextEditor::currentPart.
Definition: texteditor.cpp:153
~TextEditor() override
TextEditor::~TextEditor.
Definition: texteditor.cpp:45
void updateForm() override
TextEditor::updateForm Update the gui.
Definition: texteditor.cpp:53
void fontChanged(const QFont &font)
void setUpEditConnection()
TextEditor::setUpEditConnection Setup the connection between the widgets of this editor and the undo ...
Definition: texteditor.cpp:170
void disconnectChangeConnection()
Definition: texteditor.cpp:82
void disconnectEditConnection()
Definition: texteditor.cpp:89
QList< CustomElementPart * > currentParts() const override
Definition: texteditor.cpp:157
bool setParts(QList< CustomElementPart * >) override
Definition: texteditor.cpp:122
Definition: elementitemeditor.h:34
QList< PartText * > m_parts
Definition: texteditor.h:58
void on_m_font_pb_clicked()
TextEditor::on_m_font_pb_clicked.
Definition: texteditor.cpp:239
void on_m_color_pb_changed(const QColor &newColor)
TextEditor::on_m_color_pb_changed.
Definition: texteditor.cpp:265
Definition: autonumberingdockwidget.h:25
TextEditor(QETElementEditor *editor, PartText *text=nullptr, QWidget *parent=nullptr)
TextEditor::TextEditor Default constructor.
Definition: texteditor.cpp:31
The CustomElementPart class This abstract class represents a primitive of the visual representation o...
Definition: customelementpart.h:40
Ui::TextEditor * ui
Definition: texteditor.h:55
void colorChanged(const QColor &color)
QList< QMetaObject::Connection > m_edit_connection
Definition: texteditor.h:59
void plainTextChanged(const QString &text)
Definition: parttext.h:30
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
QIcon tr
Definition: qeticons.cpp:206
QPointer< PartText > m_text
Definition: texteditor.h:57
Definition: qetelementeditor.h:33
bool setPart(CustomElementPart *part) override
TextEditor::setPart Set the current text to edit. Set part to nullptr to clear the current text.
Definition: texteditor.cpp:103
virtual QUndoStack & undoStack() const
Definition: elementitemeditor.cpp:44