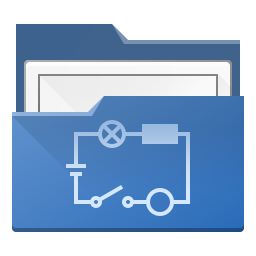 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef CONDUCTOR_SEGMENT_H
19 #define CONDUCTOR_SEGMENT_H
44 void moveX(
const qreal &);
45 void moveY(
const qreal &);
bool canMove2ndPointY(const qreal &, qreal &) const
Definition: conductorsegment.cpp:234
bool hasPreviousSegment() const
Definition: conductorsegment.cpp:479
QPointF point2
Definition: conductorsegment.h:40
void setPreviousSegment(ConductorSegment *)
Definition: conductorsegment.cpp:385
ConductorSegment * previousSegment() const
Definition: conductorsegment.cpp:421
void moveY(const qreal &)
Definition: conductorsegment.cpp:337
ConductorSegment(const ConductorSegment &)
qreal length() const
Definition: conductorsegment.cpp:505
bool canMove1stPointY(const qreal &, qreal &) const
Definition: conductorsegment.cpp:175
void setNextSegment(ConductorSegment *)
Definition: conductorsegment.cpp:396
QPointF firstPoint() const
Definition: conductorsegment.cpp:449
QET::ConductorSegmentType type() const
Definition: conductorsegment.cpp:514
bool isPoint() const
Definition: conductorsegment.cpp:519
QPointF secondPoint() const
Definition: conductorsegment.cpp:456
ConductorSegment(const QPointF &, const QPointF &, ConductorSegment *=nullptr, ConductorSegment *=nullptr)
Definition: conductorsegment.cpp:28
void moveX(const qreal &)
Definition: conductorsegment.cpp:289
bool hasNextSegment() const
Definition: conductorsegment.cpp:486
ConductorSegment * next_segment
Definition: conductorsegment.h:38
void setSecondPoint(const QPointF &)
Definition: conductorsegment.cpp:472
Definition: conductorsegment.h:25
bool isVertical() const
Definition: conductorsegment.cpp:435
bool canMove1stPointX(const qreal &, qreal &) const
Definition: conductorsegment.cpp:57
bool isFirstSegment() const
Definition: conductorsegment.cpp:409
virtual ~ConductorSegment()
Definition: conductorsegment.cpp:44
ConductorSegment * previous_segment
Definition: conductorsegment.h:37
bool isHorizontal() const
Definition: conductorsegment.cpp:442
@ Vertical
Vertical segment.
Definition: qet.h:90
bool isStatic() const
Definition: conductorsegment.cpp:404
ConductorSegmentType
Known kinds of conductor segments.
Definition: qet.h:88
QPointF middle() const
Definition: conductorsegment.cpp:493
@ Horizontal
Horizontal segment.
Definition: qet.h:89
void setFirstPoint(const QPointF &)
Definition: conductorsegment.cpp:464
QPointF point1
Definition: conductorsegment.h:39
bool isLastSegment() const
Definition: conductorsegment.cpp:414
ConductorSegment * nextSegment() const
Definition: conductorsegment.cpp:428
bool canMove2ndPointX(const qreal &, qreal &) const
Definition: conductorsegment.cpp:116