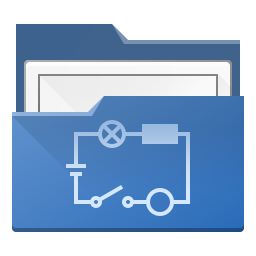 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_SLASH_TEMPLATE_COMMANDS_H
19 #define TITLEBLOCK_SLASH_TEMPLATE_COMMANDS_H
20 #define MODIFY_TITLE_BLOCK_CELL_COMMAND_ID 6378
22 #include <QUndoCommand>
43 int id()
const override;
44 bool mergeWith(
const QUndoCommand *)
override;
112 QList<TitleBlockCell *>
cells()
const;
113 void setCells(
const QList<TitleBlockCell *> &);
120 void undo()
override;
121 void redo()
override;
125 void apply(
bool =
false);
158 void undo()
override;
159 void redo()
override;
187 void undo()
override;
188 void redo()
override;
221 void undo()
override;
222 void redo()
override;
249 void undo()
override;
250 void redo()
override;
275 void undo()
override;
276 void redo()
override;
277 virtual void setCutCells(
const QList<TitleBlockCell *> &);
284 QHash<TitleBlockCell *, TitleBlockCell::TemplateCellType>
cut_cells_;
300 void undo()
override;
301 void redo()
override;
TitleBlockDimension dimension_
width/height of the column/row, which interpretation depends on type_
Definition: templatecommands.h:132
int row_span_after_
the row_span attribute of the spanning cell after the merge
Definition: templatecommands.h:204
void setIndex(int)
Definition: templatecommands.cpp:330
void redo() override
Definition: templatecommands.cpp:547
void setView(TitleBlockTemplateView *)
Definition: templatecommands.cpp:118
void redo() override
Definition: templatecommands.cpp:830
QHash< TitleBlockCell *, TitleBlockCell > erased_cells_
Existing cells impacted by the paste operation.
Definition: templatecommands.h:315
static ModifyTemplateGridCommand * deleteRow(TitleBlockTemplate *, int=-1)
Definition: templatecommands.cpp:267
~TitleBlockTemplateCommand() override
Definition: templatecommands.cpp:169
void undo() override
Definition: templatecommands.cpp:900
virtual void updateText()
Definition: templatecommands.cpp:951
void apply(const TitleBlockDimension &)
Definition: templatecommands.cpp:566
Definition: templatecommands.h:290
~ModifyTemplateDimension() override
Definition: templatecommands.cpp:475
int value
Numeric value.
Definition: dimension.h:34
Definition: templatecommands.h:140
TitleBlockDimension dimensionBefore() const
Definition: templatecommands.cpp:512
TitleBlockTemplateView * view() const
Definition: templatecommands.cpp:190
TitleBlockDimension dimensionAfter() const
Definition: templatecommands.cpp:526
int id() const override
Definition: templatecommands.cpp:48
virtual void updateText()
Definition: templatecommands.cpp:927
bool insertion_
Definition: templatecommands.h:133
bool type_
true for a row, false for a column
Definition: templatecommands.h:131
void clear()
Definition: templatecommands.cpp:125
void redo() override
Definition: templatecommands.cpp:910
int type() const
Definition: templatecommands.cpp:496
TitleBlockCell * spanning_cell_
the cell spanning over the other ones
Definition: templatecommands.h:195
int row_span_before_
the row_span attribute of the spanning cell before the merge
Definition: templatecommands.h:199
TitleBlockTemplateView * view_
This class may trigger a view update.
Definition: templatecommands.h:56
void undo() override
Definition: templatecommands.cpp:73
QHash< QString, QVariant > new_values_
values after the cell has been modified
Definition: templatecommands.h:59
ModifyTemplateGridCommand(TitleBlockTemplate *=nullptr, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:303
TitleBlockCell * spanning_cell_
the cell spanning over the other ones
Definition: templatecommands.h:226
virtual void setCutCells(const QList< TitleBlockCell * > &)
Definition: templatecommands.cpp:917
Definition: templateview.h:32
~ModifyTitleBlockCellCommand() override
Definition: templatecommands.cpp:41
TitleBlockTemplateCommand(const TitleBlockTemplateCommand &)
int col_span_before_
the col_span attribute of the spanning cell before splitting
Definition: templatecommands.h:229
int applied_col_span_before_
the applied_col_span attribute of the spanning cell before splitting
Definition: templatecommands.h:231
QSet< TitleBlockCell * > cells(bool=true) const
Definition: templatecellsset.cpp:191
ModifyTemplateDimension(TitleBlockTemplate *=nullptr, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:463
Definition: templatevisualcell.h:29
ModifyTitleBlockCellCommand(TitleBlockCell *, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:31
void undo() override
Definition: templatecommands.cpp:540
TitleBlockCell * modified_cell_
modified cell
Definition: templatecommands.h:57
void redo() override
Definition: templatecommands.cpp:405
int index() const
Definition: templatecommands.cpp:322
TitleBlockTemplateCommand(TitleBlockTemplate *=nullptr, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:159
void setType(bool)
Definition: templatecommands.cpp:504
QHash< QString, QVariant > old_values_
values before the cell is modified
Definition: templatecommands.h:58
Definition: dimension.h:26
void setDimension(const TitleBlockDimension &)
Definition: templatecommands.cpp:360
~MergeCellsCommand() override
Definition: templatecommands.cpp:634
static bool canSplit(const TitleBlockTemplateCellsSet &splitted_cells, TitleBlockTemplate *tbtemplate)
Definition: templatecommands.cpp:782
bool isValid() const
Definition: templatecommands.cpp:661
int span_state_before_
the span_state attribute of the spanning cell before splitting
Definition: templatecommands.h:232
QHash< TitleBlockCell *, TitleBlockCell * > spanner_cells_before_merge_
Definition: templatecommands.h:198
The TitleBlockTemplate class This class represents an title block template for an electric diagram....
Definition: titleblocktemplate.h:36
CutTemplateCellsCommand(TitleBlockTemplate *, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:886
QList< TitleBlockCell * > cells() const
Definition: templatecommands.cpp:337
void updateText()
Definition: templatecommands.cpp:554
bool isInsertion() const
Definition: templatecommands.cpp:383
QList< TitleBlockCell * > cells_
Cells composing the inserted/deleted row/column.
Definition: templatecommands.h:130
Definition: templatecommands.h:33
TitleBlockDimension dimension() const
Definition: templatecommands.cpp:352
TitleBlockDimension before_
Size of the row/column before it is changed.
Definition: templatecommands.h:169
void undo() override
Definition: templatecommands.cpp:398
int col_span_before_
the col_span attribute of the spanning cell before the merge
Definition: templatecommands.h:200
static ModifyTemplateGridCommand * addColumn(TitleBlockTemplate *, int=-1)
Definition: templatecommands.cpp:246
#define TITLEBLOCK_DEFAULT_COL_WIDTH
Definition: templatecommands.cpp:24
@ EmptyCell
Definition: titleblockcell.h:29
ModifyTitleBlockCellCommand(const ModifyTitleBlockCellCommand &)
virtual void addPastedCell(TitleBlockCell *, const TitleBlockCell &)
Definition: templatecommands.cpp:1040
MergeCellsCommand(const TitleBlockTemplateCellsSet &, TitleBlockTemplate *=nullptr, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:589
void refreshLayout()
Definition: templatecommands.cpp:213
TitleBlockTemplateView * view_
This class may trigger a view update.
Definition: templatecommands.h:86
~SplitCellsCommand() override
Definition: templatecommands.cpp:774
int applied_row_span_before_
the applied_row_span attribute of the spanning cell before the merge
Definition: templatecommands.h:201
PasteTemplateCellsCommand(TitleBlockTemplate *, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:937
static bool canMerge(const TitleBlockTemplateCellsSet &, TitleBlockTemplate *)
Definition: templatecommands.cpp:642
void setDimensionAfter(const TitleBlockDimension &)
Definition: templatecommands.cpp:533
int col_span
number of extra columns spanned by this cell
Definition: titleblockcell.h:64
#define TITLEBLOCK_DEFAULT_ROW_HEIGHT
Definition: templatecommands.cpp:23
bool type_
true for a row, false for a column
Definition: templatecommands.h:168
int index_
Index of the resized row/column.
Definition: templatecommands.h:167
int applied_col_span_before_
the applied_col_span attribute of the spanning cell before the merge
Definition: templatecommands.h:202
~CutTemplateCellsCommand() override
Definition: templatecommands.cpp:894
TitleBlockTemplate * titleBlockTemplate() const
Definition: templatecommands.cpp:175
static ModifyTemplateGridCommand * addRow(TitleBlockTemplate *, int=-1)
Definition: templatecommands.cpp:225
void setView(TitleBlockTemplateView *)
Definition: templatecommands.cpp:198
void undo() override
Definition: templatecommands.cpp:809
void redo() override
Definition: templatecommands.cpp:976
void setInsertion(bool)
Definition: templatecommands.cpp:390
void undo() override
Definition: templatecommands.cpp:958
@ Enabled
the cell span parameters should be applied without restriction
Definition: titleblockcell.h:35
Definition: templatecommands.h:177
void updateText()
Definition: templatecommands.cpp:412
Definition: templatecommands.h:265
QHash< TitleBlockCell *, QPair< int, int > > spans_before_
Spans before operation.
Definition: templatecommands.h:311
Definition: templatecommands.h:211
void setCell(TitleBlockCell *)
Definition: templatecommands.cpp:103
int span_state_before_
the span_state attribute of the spanning cell before the merge
Definition: templatecommands.h:203
void apply(bool=false)
Definition: templatecommands.cpp:433
int applied_row_span_before_
the applied_row_span attribute of the spanning cell before splitting
Definition: templatecommands.h:230
TitleBlockCell * cell() const
Definition: templatecommands.cpp:95
void refreshView()
Definition: templatecommands.cpp:205
static TitleBlockCell * getBottomRightCell(const TitleBlockTemplateCellsSet &)
Definition: templatecommands.cpp:713
void redo() override
Definition: templatecommands.cpp:84
void setDimensionBefore(const TitleBlockDimension &)
Definition: templatecommands.cpp:519
TitleBlockTemplate * tbtemplate_
Modified TitleBlock Template.
Definition: templatecommands.h:85
Definition: templatecommands.h:93
bool isValid() const
Definition: templatecommands.cpp:801
void setType(bool)
Definition: templatecommands.cpp:375
int row_span
number of extra rows spanned by this cell
Definition: titleblockcell.h:63
TitleBlockTemplateVisualCell * topLeftCell() const
Definition: templatecellsset.cpp:98
~ModifyTemplateGridCommand() override
Definition: templatecommands.cpp:316
virtual void addErasedCell(TitleBlockCell *, const TitleBlockCell &)
Definition: templatecommands.cpp:1048
void setIndex(int)
Definition: templatecommands.cpp:489
TitleBlockTemplateVisualCell * bottomRightCell() const
Definition: templatecellsset.cpp:129
QHash< TitleBlockCell *, TitleBlockCell::TemplateCellType > cut_cells_
Cut cells.
Definition: templatecommands.h:284
void setCells(const QList< TitleBlockCell * > &)
Definition: templatecommands.cpp:345
int index_
Index of the inserted/deleted row/column.
Definition: templatecommands.h:129
QHash< TitleBlockCell *, TitleBlockCell > pasted_cells_
Pasted cells.
Definition: templatecommands.h:313
virtual void addCell(TitleBlockCell *, const TitleBlockCell &, const TitleBlockCell &)
Definition: templatecommands.cpp:1058
int type() const
Definition: templatecommands.cpp:367
int index() const
Definition: templatecommands.cpp:481
void redo() override
Definition: templatecommands.cpp:690
void undo() override
Definition: templatecommands.cpp:669
void setTitleBlockTemplate(TitleBlockTemplate *)
Definition: templatecommands.cpp:183
ModifyTemplateGridCommand(const ModifyTemplateGridCommand &)
bool mergeWith(const QUndoCommand *) override
Definition: templatecommands.cpp:57
static ModifyTemplateGridCommand * deleteColumn(TitleBlockTemplate *, int=-1)
Definition: templatecommands.cpp:286
ModifyTemplateDimension(const ModifyTemplateDimension &)
~PasteTemplateCellsCommand() override
Definition: templatecommands.cpp:945
PasteTemplateCellsCommand(const PasteTemplateCellsCommand &)
SplitCellsCommand(const TitleBlockTemplateCellsSet &, TitleBlockTemplate *=nullptr, QUndoCommand *=nullptr)
Definition: templatecommands.cpp:744
int row_span_before_
the row_span attribute of the spanning cell before splitting
Definition: templatecommands.h:228
Definition: templatecommands.h:66
QIcon tr
Definition: qeticons.cpp:206
CutTemplateCellsCommand(const CutTemplateCellsCommand &)
#define MODIFY_TITLE_BLOCK_CELL_COMMAND_ID
Definition: templatecommands.h:20
bool isRectangle() const
Definition: templatecellsset.cpp:56
void addModification(const QString &, const QVariant &, bool=false)
Definition: templatecommands.cpp:138
int col_span_after_
the col_span attribute of the spanning cell after the merge
Definition: templatecommands.h:205
Definition: templatecellsset.h:28
Definition: titleblockcell.h:26
TitleBlockDimension after_
Size of the row/column after it is changed.
Definition: templatecommands.h:170
QSet< TitleBlockCell * > spanned_cells_
the spanned cells
Definition: templatecommands.h:227
TitleBlockTemplateView * view() const
Definition: templatecommands.cpp:110