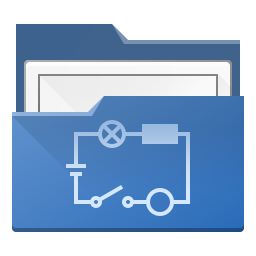 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ADDELEMENTTEXTCOMMAND_H
19 #define ADDELEMENTTEXTCOMMAND_H
21 #include <QUndoCommand>
23 #include <QDomElement>
38 QUndoCommand *parent =
nullptr);
58 QUndoCommand *parent =
nullptr);
60 const QDomElement& dom_element,
61 QUndoCommand *parent =
nullptr);
64 QList<DynamicElementTextItem *> texts_list,
65 QUndoCommand *parent =
nullptr);
89 QUndoCommand *parent =
nullptr);
109 QUndoCommand *parent =
nullptr);
112 void undo()
override;
113 void redo()
override;
129 QUndoCommand *parent =
nullptr);
132 void undo()
override;
133 void redo()
override;
148 Qt::Alignment new_alignment,
149 QUndoCommand *parent =
nullptr);
152 int id()
const override{
return 6;}
153 bool mergeWith(
const QUndoCommand *other)
override;
154 void undo()
override;
155 void redo()
override;
164 #endif // ADDELEMENTTEXTCOMMAND_H
Qt::Alignment m_new_alignment
Definition: addelementtextcommand.h:160
void redo() override
RemoveTextsGroupCommand::redo.
Definition: addelementtextcommand.cpp:251
AddTextsGroupCommand(Element *element, QString groupe_name, QUndoCommand *parent=nullptr)
AddTextsGroupCommand::AddTextsGroupCommand.
Definition: addelementtextcommand.cpp:88
QList< DynamicElementTextItem * > m_deti_list
Definition: addelementtextcommand.h:74
The DynamicElementTextItem class This class provide a simple text field of element who can be added o...
Definition: dynamicelementtextitem.h:39
void redo() override
RemoveTextFromGroupCommand::redo.
Definition: addelementtextcommand.cpp:372
QPointer< Element > m_element
Definition: addelementtextcommand.h:72
int id() const override
Definition: addelementtextcommand.h:152
~RemoveTextFromGroupCommand() override
RemoveTextFromGroupCommand::~RemoveTextFromGroupCommand Destructor.
Definition: addelementtextcommand.cpp:350
QList< QPointer< DynamicElementTextItem > > m_text_list
Definition: addelementtextcommand.h:98
void undo() override
RemoveTextsGroupCommand::undo.
Definition: addelementtextcommand.cpp:234
AlignmentTextsGroupCommand(ElementTextItemGroup *group, Qt::Alignment new_alignment, QUndoCommand *parent=nullptr)
AlignmentTextsGroupCommand::AlignmentTextsGroupCommand.
Definition: addelementtextcommand.cpp:388
AddTextToGroupCommand(DynamicElementTextItem *text, ElementTextItemGroup *group, QUndoCommand *parent=nullptr)
AddTextToGroupCommand::AddTextToGroupCommand.
Definition: addelementtextcommand.cpp:275
~RemoveTextsGroupCommand() override
RemoveTextsGroupCommand::~RemoveTextsGroupCommand.
Definition: addelementtextcommand.cpp:228
QPointer< ElementTextItemGroup > m_group
Definition: addelementtextcommand.h:73
bool mergeWith(const QUndoCommand *other) override
AlignmentTextsGroupCommand::mergeWith Try to merge this command with other command.
Definition: addelementtextcommand.cpp:422
QPointer< ElementTextItemGroup > m_group
Definition: addelementtextcommand.h:158
void redo() override
AddTextToGroupCommand::redo.
Definition: addelementtextcommand.cpp:312
QPointer< Element > m_element
Definition: addelementtextcommand.h:118
void redo() override
AddElementTextCommand::redo.
Definition: addelementtextcommand.cpp:72
The AddTextsGroupCommand class Manage the adding of a texts group.
Definition: addelementtextcommand.h:54
~AddElementTextCommand() override
AddElementTextCommand::~AddElementTextCommand.
Definition: addelementtextcommand.cpp:50
QHash< DynamicElementTextItem *, QPointF > m_texts_pos
Definition: addelementtextcommand.h:161
void undo() override
AddTextsGroupCommand::undo.
Definition: addelementtextcommand.cpp:156
RemoveTextsGroupCommand(Element *element, ElementTextItemGroup *group, QUndoCommand *parent=nullptr)
RemoveTextsGroupCommand::RemoveTextsGroupCommand.
Definition: addelementtextcommand.cpp:212
RemoveTextFromGroupCommand(DynamicElementTextItem *text, ElementTextItemGroup *group, QUndoCommand *parent=nullptr)
RemoveTextFromGroupCommand::RemoveTextFromGroupCommand.
Definition: addelementtextcommand.cpp:334
QList< DynamicElementTextItem * > texts() const
ElementTextItemGroup::texts.
Definition: elementtextitemgroup.cpp:330
void removeDynamicTextItem(DynamicElementTextItem *deti)
Element::removeDynamicTextItem Remove deti, no matter if is a child of this element or a child of a g...
Definition: element.cpp:1287
void undo() override
AlignmentTextsGroupCommand::undo.
Definition: addelementtextcommand.cpp:439
void addDynamicTextItem(DynamicElementTextItem *deti=nullptr)
Element::addDynamiqueTextItem Add deti as a dynamic text item of this element, deti is reparented to ...
Definition: element.cpp:1264
The AlignmentTextsGroupCommand class.
Definition: addelementtextcommand.h:145
void undo() override
RemoveTextFromGroupCommand::undo.
Definition: addelementtextcommand.cpp:363
QPointer< Element > m_element
Definition: addelementtextcommand.h:96
The RemoveTextsGroupCommand class Manage the removinf of a texts group.
Definition: addelementtextcommand.h:85
QPointer< Element > m_element
Definition: addelementtextcommand.h:138
QDomElement m_dom_element
Definition: addelementtextcommand.h:75
Element * m_element
Definition: addelementtextcommand.h:45
QString m_name
Definition: addelementtextcommand.h:76
QPointer< ElementTextItemGroup > m_group
Definition: addelementtextcommand.h:97
The RemoveTextFromGroupCommand class.
Definition: addelementtextcommand.h:125
void undo() override
AddTextToGroupCommand::undo.
Definition: addelementtextcommand.cpp:303
QPointer< ElementTextItemGroup > m_group
Definition: addelementtextcommand.h:117
void redo() override
AddTextsGroupCommand::redo.
Definition: addelementtextcommand.cpp:165
The ElementTextItemGroup class This class represent a group of element text Texts in the group can be...
Definition: elementtextitemgroup.h:36
AddElementTextCommand(Element *element, DynamicElementTextItem *deti, QUndoCommand *parent=nullptr)
AddElementTextCommand::AddElementTextCommand.
Definition: addelementtextcommand.cpp:37
QPointer< DynamicElementTextItem > m_text
Definition: addelementtextcommand.h:136
void undo() override
AddElementTextCommand::undo.
Definition: addelementtextcommand.cpp:62
QPointer< ElementTextItemGroup > m_group
Definition: addelementtextcommand.h:137
~AddTextToGroupCommand() override
AddTextToGroupCommand::~AddTextToGroupCommand Destructor.
Definition: addelementtextcommand.cpp:290
~AlignmentTextsGroupCommand() override
AlignmentTextsGroupCommand::~AlignmentTextsGroupCommand Destructor.
Definition: addelementtextcommand.cpp:413
ElementTextItemGroup * parentGroup() const
DynamicElementTextItem::parentGroup.
Definition: dynamicelementtextitem.cpp:236
~AddTextsGroupCommand() override
AddTextsGroupCommand::~AddTextsGroupCommand Destructor.
Definition: addelementtextcommand.cpp:150
DynamicElementTextItem * m_text
Definition: addelementtextcommand.h:46
Qt::Alignment m_previous_alignment
Definition: addelementtextcommand.h:159
bool m_first_undo
Definition: addelementtextcommand.h:77
QIcon tr
Definition: qeticons.cpp:206
The AddTextToGroupCommand class.
Definition: addelementtextcommand.h:105
void redo() override
AlignmentTextsGroupCommand::redo.
Definition: addelementtextcommand.cpp:460
QList< DynamicElementTextItem * > dynamicTextItems() const
Element::dynamicTextItems.
Definition: element.cpp:1316
QPointer< DynamicElementTextItem > m_text
Definition: addelementtextcommand.h:116
The AddElementTextCommand class Manage the adding of element text.
Definition: addelementtextcommand.h:34