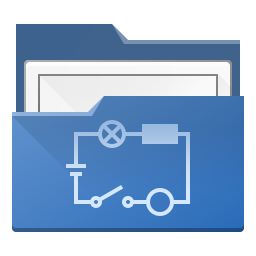 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_SLASH_HELPER_CELL_H
19 #define TITLEBLOCK_SLASH_HELPER_CELL_H
27 class HelperCell :
public QGraphicsObject,
public QGraphicsLayoutItem {
29 Q_INTERFACES(QGraphicsLayoutItem)
49 QSizeF
sizeHint(Qt::SizeHint,
const QSizeF & = QSizeF())
const override;
51 void paint(QPainter *,
const QStyleOptionGraphicsItem *, QWidget * =
nullptr)
override;
53 virtual void setActions(
const QList<QAction *> &);
54 virtual QList<QAction *>
actions()
const;
55 virtual void setLabel(
const QString &text,
bool =
true);
@ RelativeToTotalLength
the length is just a fraction of the total available length
Definition: qet.h:147
virtual QList< QAction * > actions() const
Definition: helpercell.cpp:117
void mouseDoubleClickEvent(QGraphicsSceneMouseEvent *) override
Definition: helpercell.cpp:150
Definition: helpercell.h:27
QColor background_color
Background color when rendering this cell.
Definition: helpercell.h:40
TitleBlockColumnLength
enum used to specify the type of a length
Definition: qet.h:145
void paint(QPainter *, const QStyleOptionGraphicsItem *, QWidget *=nullptr) override
Definition: helpercell.cpp:77
void doubleClicked(HelperCell *)
HelperCell(QGraphicsItem *=nullptr)
Definition: helpercell.cpp:24
HelperCell(const HelperCell &)
~HelperCell() override
Definition: helpercell.cpp:40
@ Absolute
the length is absolute and should be applied as is
Definition: qet.h:146
void contextMenuEvent(QGraphicsSceneContextMenuEvent *) override
Definition: helpercell.cpp:136
QList< QAction * > actions_
List of actions displayed by the context menu.
Definition: helpercell.h:66
void setGeometry(const QRectF &) override
Definition: helpercell.cpp:48
QRectF boundingRect() const override
Definition: helpercell.cpp:67
QString label
Label displayed in this cell.
Definition: helpercell.h:42
virtual void setActions(const QList< QAction * > &)
Definition: helpercell.cpp:110
QColor foreground_color
Text color when rendering this cell.
Definition: helpercell.h:41
virtual void setLabel(const QString &text, bool=true)
Definition: helpercell.cpp:125
virtual void setType(QET::TitleBlockColumnLength)
Definition: helpercell.cpp:94
int index
Index of this cell.
Definition: helpercell.h:44
@ Horizontal
Horizontal segment.
Definition: qet.h:89
QSizeF sizeHint(Qt::SizeHint, const QSizeF &=QSizeF()) const override
Definition: helpercell.cpp:59
void contextMenuTriggered(HelperCell *)
@ RelativeToRemainingLength
the length is just a fraction of the length that is still available when other types of lengths have ...
Definition: qet.h:148
Qt::Orientation orientation
Orientation of this cell.
Definition: helpercell.h:43