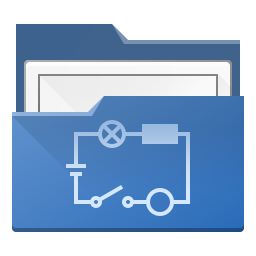 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTS_COLLECTION_CACHE_H
19 #define ELEMENTS_COLLECTION_CACHE_H
21 #include <QSqlDatabase>
51 const QUuid &uuid = QUuid::createUuid());
53 const QUuid &uuid = QUuid::createUuid());
bool fetchElement(ElementsLocation &location)
ElementsCollectionCache::fetchElement Retrieve the data for a given element, using the cache if avail...
Definition: elementscollectioncache.cpp:161
QString pixmap_storage_format_
Storage format for cached pixmaps.
Definition: elementscollectioncache.h:63
QString locale_
Locale to be used when dealing with names.
Definition: elementscollectioncache.h:62
bool fetchNameFromCache(const QString &path, const QUuid &uuid)
ElementsCollectionCache::fetchNameFromCache Retrieve the name for an element, given its path and uuid...
Definition: elementscollectioncache.cpp:235
QString locale() const
Definition: elementscollectioncache.cpp:126
bool cacheName(const QString &path, const QUuid &uuid=QUuid::createUuid())
ElementsCollectionCache::cacheName Cache the current (i.e. last retrieved) name The cache entry will ...
Definition: elementscollectioncache.cpp:295
QSqlDatabase cache_db_
Object providing access to the SQLite database this cache relies on.
Definition: elementscollectioncache.h:57
Definition: elementscollectioncache.h:31
bool fetchData(const ElementsLocation &)
Definition: elementscollectioncache.cpp:211
QSqlQuery * insert_name_
Prepared statement to insert names into the cache.
Definition: elementscollectioncache.h:60
QString name() const
Definition: elementscollectioncache.cpp:193
QUuid uuid() const
ElementsLocation::uuid.
Definition: elementslocation.cpp:737
QSqlQuery * select_pixmap_
Prepared statement to fetch pixmaps from the cache.
Definition: elementscollectioncache.h:59
QString pixmapStorageFormat() const
Definition: elementscollectioncache.cpp:148
QSqlQuery * select_name_
Prepared statement to fetch names from the cache.
Definition: elementscollectioncache.h:58
~ElementsCollectionCache() override
Definition: elementscollectioncache.cpp:107
bool fetchPixmapFromCache(const QString &path, const QUuid &uuid)
ElementsCollectionCache::fetchPixmapFromCache Retrieve the pixmap for an element, given its path and ...
Definition: elementscollectioncache.cpp:264
QPixmap current_pixmap_
Last pixmap fetched.
Definition: elementscollectioncache.h:65
QString current_name_
Last name fetched.
Definition: elementscollectioncache.h:64
void setLocale(const QString &)
Definition: elementscollectioncache.cpp:119
QSqlQuery * insert_pixmap_
Prepared statement to insert pixmaps into the cache.
Definition: elementscollectioncache.h:61
bool isProject() const
ElementsLocation::isProject.
Definition: elementslocation.cpp:459
static ElementFactory * Instance()
Definition: elementfactory.h:38
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
ElementsCollectionCache(const QString &database_path, QObject *=nullptr)
Definition: elementscollectioncache.cpp:32
QPixmap pixmap() const
Definition: elementscollectioncache.cpp:200
QString toString() const
ElementsLocation::toString.
Definition: elementslocation.cpp:396
bool setPixmapStorageFormat(const QString &)
Definition: elementscollectioncache.cpp:136
bool cachePixmap(const QString &path, const QUuid &uuid=QUuid::createUuid())
ElementsCollectionCache::cachePixmap Cache the current (i.e. last retrieved) pixmap.
Definition: elementscollectioncache.cpp:318