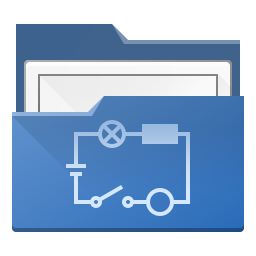 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_TEMPLATE_H
19 #define TITLEBLOCK_TEMPLATE_H
76 bool insertRow(
int,
const QList<TitleBlockCell *> &,
int = -1);
77 QList<TitleBlockCell *>
takeRow(
int);
83 const QList<TitleBlockCell *> &,
int = -1);
90 QHash<TitleBlockCell *, QPair<int, int> >
getAllSpans()
const;
94 const QString & =
"svg",
95 const QString & =
"xml");
99 bool renameLogo(
const QString &,
const QString &);
101 QList<QString>
logos()
const;
102 QString
logoType(
const QString &)
const;
103 QSvgRenderer *
vectorLogo(
const QString &)
const;
115 const QRect &)
const;
127 bool loadLogos(
const QDomElement &,
bool =
false);
134 void saveLogo(
const QString &, QDomElement &)
const;
146 int lengthRange(
int,
int,
const QList<int> &)
const;
154 const QRectF &)
const;
~TitleBlockTemplate() override
TitleBlockTemplate::~TitleBlockTemplate Destructor.
Definition: titleblocktemplate.cpp:40
void applyCellSpan(TitleBlockCell *)
TitleBlockTemplate::applyCellSpan Ensure the spans of the provided cell are applied within the grid s...
Definition: titleblocktemplate.cpp:1983
QList< TitleBlockCell * > takeColumn(int)
TitleBlockTemplate::takeColumn Removes the column at index i.
Definition: titleblocktemplate.cpp:1118
static const double sheetHeight
Definition: createdxf.h:112
QHash< QString, QString > type_logos_
type_logos_ : Logos types (e.g. "png", "jpeg", "svg")
Definition: titleblocktemplate.h:185
int rowsCount() const
TitleBlockTemplate::rowsCount.
Definition: titleblocktemplate.cpp:814
QList< QString > keys(KeyOrder=None) const
Definition: diagramcontext.cpp:49
@ RelativeToTotalLength
the length is just a fraction of the total available length
Definition: qet.h:147
int height() const
TitleBlockTemplate::height.
Definition: titleblocktemplate.cpp:983
bool removeLogo(const QString &)
TitleBlockTemplate::removeLogo.
Definition: titleblocktemplate.cpp:1343
TitleBlockTemplate(const TitleBlockTemplate &)
static double yScale
Definition: createdxf.h:114
bool hadjust
Whether to reduce the font size if the text does not fit in the cell.
Definition: titleblockcell.h:75
TitleBlockTemplate * clone() const
TitleBlockTemplate::clone.
Definition: titleblocktemplate.cpp:190
void applyCellSpans()
TitleBlockTemplate::applyCellSpans Forget any previously applied span, then apply again all spans def...
Definition: titleblocktemplate.cpp:1905
int value
Numeric value.
Definition: dimension.h:34
bool loadLogos(const QDomElement &, bool=false)
TitleBlockTemplate::loadLogos Import the logos from a given XML titleblock template.
Definition: titleblocktemplate.cpp:273
void renderDxf(QRectF &, const DiagramContext &, int, QString &, int) const
TitleBlockTemplate::renderDxf Render the titleblock in DXF.
Definition: titleblocktemplate.cpp:1523
bool isEmpty() const
Definition: nameslist.cpp:73
QET::TitleBlockColumnLength type
Kind of length.
Definition: dimension.h:33
QString name() const
TitleBlockTemplate::name.
Definition: titleblocktemplate.cpp:727
void addRow(int=-1)
TitleBlockTemplate::addRow Add a new 25px-wide row at the provided index.
Definition: titleblocktemplate.cpp:1015
QString finalTextForCell(const TitleBlockCell &, const DiagramContext &) const
TitleBlockTemplate::finalTextForCell.
Definition: titleblocktemplate.cpp:1660
QString interpreteVariables(const QString &, const DiagramContext &) const
TitleBlockTemplate::interpreteVariables.
Definition: titleblocktemplate.cpp:1686
bool loadFromXmlFile(const QString &)
TitleBlockTemplate::loadFromXmlFile Load a titleblock template from an XML file.
Definition: titleblocktemplate.cpp:90
int columnTypeCount(QET::TitleBlockColumnLength)
TitleBlockTemplate::columnTypeCount.
Definition: titleblocktemplate.cpp:902
bool moveRow(int, int)
TitleBlockTemplate::moveRow Move a row within this template.
Definition: titleblocktemplate.cpp:998
TitleBlockDimension columnDimension(int)
TitleBlockTemplate::columnDimension.
Definition: titleblocktemplate.cpp:779
bool insertRow(int, const QList< TitleBlockCell * > &, int=-1)
TitleBlockTemplate::insertRow.
Definition: titleblocktemplate.cpp:1029
void saveInformation(QDomElement &) const
TitleBlockTemplate::saveInformation Export this template's extra information.
Definition: titleblocktemplate.cpp:503
void exportCellToXml(TitleBlockCell *, QDomElement &) const
TitleBlockTemplate::exportCellToXml.
Definition: titleblocktemplate.cpp:179
void forgetSpanning()
TitleBlockTemplate::forgetSpanning Set the spanner_cell attribute of every cell to 0.
Definition: titleblocktemplate.cpp:1868
void rowColsChanged()
TitleBlockTemplate::rowColsChanged Take care of consistency and span-related problematics when adding...
Definition: titleblocktemplate.cpp:2030
QHash< QString, QString > storage_logos_
storage_logos_ : Logos applied storage type (e.g. "xml" or "base64")
Definition: titleblocktemplate.h:180
TitleBlockColumnLength
enum used to specify the type of a length
Definition: qet.h:145
TitleBlockTemplate(QObject *=nullptr)
TitleBlockTemplate::TitleBlockTemplate Constructor.
Definition: titleblocktemplate.cpp:31
void setAllSpans(const QHash< TitleBlockCell *, QPair< int, int > > &)
TitleBlockTemplate::setAllSpans Restore a set of span parameters.
Definition: titleblocktemplate.cpp:1221
void initCells()
TitleBlockTemplate::initCells Initialize the internal cells grid with the row and column counts....
Definition: titleblocktemplate.cpp:709
QList< TitleBlockCell * > createRow()
TitleBlockTemplate::createRow.
Definition: titleblocktemplate.cpp:1064
QList< TitleBlockCell * > registered_cells_
registered_cells_ : Cells objects created rattached to this template, but not mandatorily used
Definition: titleblocktemplate.h:205
void setColumnDimension(int, const TitleBlockDimension &)
TitleBlockTemplate::setColumnDimension Set the width of a column.
Definition: titleblocktemplate.cpp:793
bool saveToXmlElement(QDomElement &) const
TitleBlockTemplate::saveToXmlElement Save the title block template as XML.
Definition: titleblocktemplate.cpp:161
QString name_
name_ : name identifying the Title Block Template within its parent collection
Definition: titleblocktemplate.h:170
@ TextCell
Definition: titleblockcell.h:30
void setInformation(const QString &)
TitleBlockTemplate::setInformation.
Definition: titleblocktemplate.cpp:743
Definition: dimension.h:26
int lengthRange(int, int, const QList< int > &) const
TitleBlockTemplate::lengthRange.
Definition: titleblocktemplate.cpp:2044
static QFont fontForCell(const TitleBlockCell &)
TitleBlockTemplate::fontForCell.
Definition: titleblocktemplate.cpp:80
void renderTextCell(QPainter &, const QString &, const TitleBlockCell &, const QRectF &) const
TitleBlockTemplate::renderTextCell This method uses a painter to render the text of a cell into the c...
Definition: titleblocktemplate.cpp:1738
bool renameLogo(const QString &, const QString &)
TitleBlockTemplate::renameLogo Rename the logo_name logo to new_name.
Definition: titleblocktemplate.cpp:1367
QSet< TitleBlockCell * > spannedCells(const TitleBlockCell *, bool=false) const
TitleBlockTemplate::spannedCells.
Definition: titleblocktemplate.cpp:1161
void renderTextCellDxf(QString &, const QString &, const TitleBlockCell &, qreal, qreal, qreal, qreal, int) const
TitleBlockTemplate::renderTextCellDxf.
Definition: titleblocktemplate.cpp:1792
The TitleBlockTemplate class This class represents an title block template for an electric diagram....
Definition: titleblocktemplate.h:36
NamesList value
Text displayed by the cell.
Definition: titleblockcell.h:70
TitleBlockCell * cell(int, int) const
TitleBlockTemplate::cell.
Definition: titleblocktemplate.cpp:1143
@ Disabled
the cell span parameters should not applied at all
Definition: titleblockcell.h:34
void renderCell(QPainter &, const TitleBlockCell &, const DiagramContext &, const QRect &) const
TitleBlockTemplate::renderCell Render a titleblock cell.
Definition: titleblocktemplate.cpp:1609
QString toShortString() const
Definition: dimension.cpp:49
bool addLogoFromFile(const QString &, const QString &=QString())
TitleBlockTemplate::addLogoFromFile.
Definition: titleblocktemplate.cpp:1293
@ EmptyCell
Definition: titleblockcell.h:29
TemplateCellType type() const
Definition: titleblockcell.cpp:29
@ Absolute
the length is absolute and should be applied as is
Definition: qet.h:146
QList< TitleBlockCell * > createCellsList(int)
TitleBlockTemplate::createCellsList.
Definition: titleblocktemplate.cpp:68
QHash< TitleBlockCell *, QPair< int, int > > getAllSpans() const
TitleBlockTemplate::getAllSpans Export the span parameters of all cell in the current grid.
Definition: titleblocktemplate.cpp:1199
NamesList label
Label displayed by the cell.
Definition: titleblockcell.h:71
TitleBlockCell * createCell(const TitleBlockCell *=nullptr)
TitleBlockTemplate::createCell Create a new cell and associate it with this template,...
Definition: titleblocktemplate.cpp:52
QString name(const QString &=QString()) const
Definition: nameslist.cpp:231
void saveCell(TitleBlockCell *, QDomElement &, bool=false) const
TitleBlockTemplate::saveCell Export a specific cell as XML.
Definition: titleblocktemplate.cpp:611
bool loadFromXmlElement(const QDomElement &)
TitleBlockTemplate::loadFromXmlElement.
Definition: titleblocktemplate.cpp:117
bool writeXmlFile(QDomDocument &xml_doc, const QString &filepath, QString *error_message=nullptr)
Definition: qet.cpp:584
void loadInformation(const QDomElement &)
TitleBlockTemplate::loadInformation Import text informations from a given XML title block template.
Definition: titleblocktemplate.cpp:255
void setLogoStorage(const QString &, const QString &)
TitleBlockTemplate::setLogoStorage Set the kind of storage for the logo_name logo.
Definition: titleblocktemplate.cpp:1394
bool display_label
Whether to display the label or not.
Definition: titleblockcell.h:72
@ Enabled
the cell span parameters should be applied without restriction
Definition: titleblockcell.h:35
QHash< QString, QPixmap > bitmap_logos_
bitmap_logos_ : Pixmaps for bitmap logos
Definition: titleblocktemplate.h:193
void saveLogos(QDomElement &) const
TitleBlockTemplate::saveLogos Export this template's logos as XML.
Definition: titleblocktemplate.cpp:519
void parseRows(const QString &)
TitleBlockTemplate::parseRows Parse the rows heights.
Definition: titleblocktemplate.cpp:382
QList< QString > logos() const
TitleBlockTemplate::logos.
Definition: titleblocktemplate.cpp:1405
bool loadLogo(const QDomElement &)
TitleBlockTemplate::loadLogo Import the logo from a given XML logo description.
Definition: titleblocktemplate.cpp:312
static void drawRectangle(const QString &filepath, double, double, double, double, const int &colorcode)
Definition: createdxf.cpp:565
static double xScale
Definition: createdxf.h:113
bool saveToXmlFile(const QString &)
TitleBlockTemplate::saveToXmlFile Save the title block template into an XML file.
Definition: titleblocktemplate.cpp:141
@ LogoCell
Definition: titleblockcell.h:31
QList< int > rows_heights_
rows heights – simple integers
Definition: titleblocktemplate.h:195
int columnTypeTotal(QET::TitleBlockColumnLength)
TitleBlockTemplate::columnTypeTotal.
Definition: titleblocktemplate.cpp:917
bool saveLogoToFile(const QString &, const QString &)
TitleBlockTemplate::saveLogoToFile.
Definition: titleblocktemplate.cpp:1322
void applyRowColNums()
TitleBlockTemplate::applyRowColNums Ensure all cells have the right col+row numbers.
Definition: titleblocktemplate.cpp:2016
int rowDimension(int)
TitleBlockTemplate::rowDimension.
Definition: titleblocktemplate.cpp:752
bool addLogo(const QString &, QByteArray *, const QString &="svg", const QString &="xml")
TitleBlockTemplate::addLogo.
Definition: titleblocktemplate.cpp:1239
int minimumWidth()
Definition: titleblocktemplate.cpp:932
QSvgRenderer * vectorLogo(const QString &) const
TitleBlockTemplate::vectorLogo.
Definition: titleblocktemplate.cpp:1430
void render(QPainter &, const DiagramContext &, int) const
TitleBlockTemplate::render Render the titleblock.
Definition: titleblocktemplate.cpp:1461
void setRowDimension(int, const TitleBlockDimension &)
TitleBlockTemplate::setRowDimension Set the height of a row.
Definition: titleblocktemplate.cpp:766
QHash< QString, QByteArray > data_logos_
data_logos_ : Logos raw data
Definition: titleblocktemplate.h:175
QHash< QString, QSvgRenderer * > vector_logos_
vector_logos_ : Rendered objects for vector logos
Definition: titleblocktemplate.h:189
static QFont diagramTextsFont(qreal=-1.0)
QETApp::diagramTextsFont The font to use By default the font is "sans Serif" and size 9.
Definition: qetapp.cpp:1111
QList< TitleBlockDimension > columns_width_
columns_width_ : columns widths –
Definition: titleblocktemplate.h:200
bool attributeIsAnInteger(const QDomElement &, const QString &, int *=nullptr)
Definition: qet.cpp:210
static void drawTextAligned(const QString &fileName, const QString &text, double x, double y, double height, double rotation, double oblique, int hAlign, int vAlign, double xAlign, double xScale, int colour, bool leftAlign=false, float scale=0)
Definition: createdxf.cpp:781
QPixmap bitmapLogo(const QString &) const
TitleBlockTemplate::bitmapLogo.
Definition: titleblocktemplate.cpp:1444
QString logoType(const QString &) const
TitleBlockTemplate::logoType.
Definition: titleblocktemplate.cpp:1416
QList< int > rowsHeights() const
TitleBlockTemplate::rowsHeights.
Definition: titleblocktemplate.cpp:893
int maximumWidth()
TitleBlockTemplate::maximumWidth.
Definition: titleblocktemplate.cpp:957
void saveCells(QDomElement &) const
TitleBlockTemplate::saveCells Export this template's cells as XML (without the grid-related informati...
Definition: titleblocktemplate.cpp:591
bool loadGrid(const QDomElement &)
Definition: titleblocktemplate.cpp:350
QString information() const
TitleBlockTemplate::information.
Definition: titleblocktemplate.cpp:735
QStringList listOfVariables()
TitleBlockTemplate::listOfVariables Get list of variables.
Definition: titleblocktemplate.cpp:1705
bool moveColumn(int, int)
TitleBlockTemplate::moveColumn Move the column at index "from" to index "to".
Definition: titleblocktemplate.cpp:1075
@ Restricted
the cell span parameters should be applied with some restrictions
Definition: titleblockcell.h:36
void parseColumns(const QString &)
TitleBlockTemplate::parseColumns Parse the columns widths.
Definition: titleblocktemplate.cpp:416
QString information_
Definition: titleblocktemplate.h:171
bool checkCell(const QDomElement &, TitleBlockCell **=nullptr)
TitleBlockTemplate::checkCell Load the essential attributes of a cell: row and column indices and spa...
Definition: titleblocktemplate.cpp:648
int columnsCount() const
TitleBlockTemplate::columnsCount.
Definition: titleblocktemplate.cpp:806
void addColumn(int=-1)
TitleBlockTemplate::addColumn Add a new 50px-wide column at the provided index.
Definition: titleblocktemplate.cpp:1090
int font_size
Font size the text should be rendered with.
Definition: titleblockcell.h:74
void saveGrid(QDomElement &) const
TitleBlockTemplate::saveGrid Export this template's cells grid as XML.
Definition: titleblocktemplate.cpp:567
QIcon tr
Definition: qeticons.cpp:206
QList< QList< TitleBlockCell * > > cells_
Cells grid.
Definition: titleblocktemplate.h:206
void saveLogo(const QString &, QDomElement &) const
TitleBlockTemplate::saveLogo Export a specific logo as XML.
Definition: titleblocktemplate.cpp:537
bool insertColumn(const TitleBlockDimension &, const QList< TitleBlockCell * > &, int=-1)
TitleBlockTemplate::insertColumn.
Definition: titleblocktemplate.cpp:1102
QList< TitleBlockCell * > createColumn()
TitleBlockTemplate::createColumn.
Definition: titleblocktemplate.cpp:1133
Definition: diagramcontext.h:56
QString logo_reference
Logo displayed by this cell, it it is a logo cell.
Definition: titleblockcell.h:76
Definition: titleblockcell.h:26
QList< int > columnsWidth(int) const
TitleBlockTemplate::columnsWidth.
Definition: titleblocktemplate.cpp:823
int width(int)
TitleBlockTemplate::width.
Definition: titleblocktemplate.cpp:971
QList< TitleBlockCell * > takeRow(int)
TitleBlockTemplate::takeRow Removes the row at index i.
Definition: titleblocktemplate.cpp:1048
void loadCell(const QDomElement &)
TitleBlockTemplate::loadCell Load a cell into this template.
Definition: titleblocktemplate.cpp:491
int alignment
Where the label+text should be displayed within the visual cell.
Definition: titleblockcell.h:73
@ RelativeToRemainingLength
the length is just a fraction of the length that is still available when other types of lengths have ...
Definition: qet.h:148
bool loadCells(const QDomElement &)
TitleBlockTemplate::loadCells Analyze an XML element, looking for grid cells. The grid cells are chec...
Definition: titleblocktemplate.cpp:469
bool checkCellSpan(TitleBlockCell *)
TitleBlockTemplate::checkCellSpan Check whether a given cell can be spanned according to its row_span...
Definition: titleblocktemplate.cpp:1927
@ DecreasingLength
Definition: diagramcontext.h:61