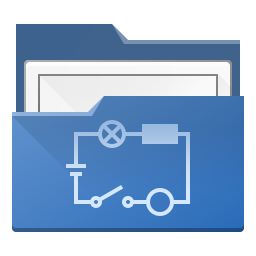 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENTCOLLECTIONHANDLER_H
19 #define ELEMENTCOLLECTIONHANDLER_H
58 const QString& rename = QString());
62 const QString& rename = QString());
81 const QString& rename = QString());
85 const QString& rename = QString());
128 #endif // ELEMENTCOLLECTIONHANDLER_H
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
~ElementCollectionHandler()
Definition: elementcollectionhandler.cpp:307
bool createDir(const QString &path, const QString &name, const NamesList &name_list)
XmlElementCollection::createDir Create a child directorie at path path with the name name....
Definition: xmlelementcollection.cpp:587
ElementsLocation copy() override
Definition: elementcollectionhandler.cpp:167
Definition: renamedialog.h:29
ECHSXmlToFile(ElementsLocation &source, ElementsLocation &destination)
Definition: elementcollectionhandler.cpp:163
The ECHStrategy class Abstract class for manage copy of directory or element from a collection to ano...
Definition: elementcollectionhandler.h:32
XmlElementCollection * embeddedElementCollection() const
QETProject::embeddedCollection.
Definition: qetproject.cpp:236
ElementsLocation m_destination
Definition: elementcollectionhandler.h:39
ElementsLocation copy(ElementsLocation &source, ElementsLocation &destination)
ElementCollectionHandler::copy Copy the content of collection represented by source to the collection...
Definition: elementcollectionhandler.cpp:321
QString fileName() const
ElementLocation::fileName.
Definition: elementslocation.cpp:793
ElementsLocation copyElement(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString())
Definition: elementcollectionhandler.cpp:252
ECHStrategy(ElementsLocation &source, ElementsLocation &destination)
Definition: elementcollectionhandler.cpp:28
ElementsLocation copy(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString(), bool deep_copy=true)
XmlElementCollection::copy Copy the content represented by source (an element or a directory) to dest...
Definition: xmlelementcollection.cpp:545
QStringList directoriesNames(const QDomElement &parent_element) const
XmlElementCollection::directoriesNames.
Definition: xmlelementcollection.cpp:226
bool isWritable() const
ElementsLocation::isWritable.
Definition: elementslocation.cpp:499
ElementsLocation copyDirectory(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString())
Definition: elementcollectionhandler.cpp:205
ECHStrategy * m_strategy
Definition: elementcollectionhandler.h:125
The XmlElementCollection class This class represent a collection of elements stored to xml.
Definition: xmlelementcollection.h:34
bool isFileSystem() const
ElementsLocation::isFileSystem.
Definition: elementslocation.cpp:428
ElementsLocation copy() override
Definition: elementcollectionhandler.cpp:42
bool importFromProject(QETProject *project, ElementsLocation &location)
ElementCollectionHandler::importFromProject Import the element represented by location to the embedde...
Definition: elementcollectionhandler.cpp:405
bool isElement() const
ElementsLocation::isElement.
Definition: elementslocation.cpp:412
QStringList elementsNames(const QDomElement &parent_element) const
XmlElementCollection::elementsNames.
Definition: xmlelementcollection.cpp:269
The ElementCollectionHandler class Provide several method to copy element or directory from a collect...
Definition: elementcollectionhandler.h:109
ElementsLocation copyDirectory(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString())
Definition: elementcollectionhandler.cpp:80
ElementsLocation parent() const
ElementsLocation::parent.
Definition: elementslocation.cpp:346
virtual ~ECHStrategy()
Definition: elementcollectionhandler.cpp:34
ECHSToXml(ElementsLocation &source, ElementsLocation &destination)
Definition: elementcollectionhandler.cpp:272
QDomElement xml() const
ElementsLocation::xml.
Definition: elementslocation.cpp:575
@ Rename
the target has to be renamed
Definition: qet.h:133
ElementsLocation m_source
Definition: elementcollectionhandler.h:39
bool addToPath(const QString &)
ElementsLocation::addToPath Add a string to the actual path of this location.
Definition: elementslocation.cpp:320
bool isDirectory() const
ElementsLocation::isDirectory.
Definition: elementslocation.cpp:420
QString projectCollectionPath() const
ElementsLocation::projectCollectionPath.
Definition: elementslocation.cpp:176
ElementsLocation copyElement(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString())
Definition: elementcollectionhandler.cpp:134
ElementsLocation createDir(ElementsLocation &parent, const QString &name, const NamesList &name_list)
ElementCollectionHandler::createDir Create a directorie with name as child of parent....
Definition: elementcollectionhandler.cpp:344
QString fileSystemPath() const
ElementsLocation::fileSystemPath.
Definition: elementslocation.cpp:193
bool writeXmlFile(QDomDocument &xml_doc, const QString &filepath, QString *error_message=nullptr)
Definition: qet.cpp:584
QString newName() const
Definition: renamedialog.h:36
bool isProject() const
ElementsLocation::isProject.
Definition: elementslocation.cpp:459
bool setNames(ElementsLocation &location, const NamesList &name_list)
ElementCollectionHandler::setNames Set the names stored in name_list as the names of the item represe...
Definition: elementcollectionhandler.cpp:454
QDomElement directory(const QString &path) const
XmlElementCollection::directory.
Definition: xmlelementcollection.cpp:309
QString collectionPath(bool protocol=true) const
ElementsLocation::collectionPath Return the path of the represented element relative to collection if...
Definition: elementslocation.cpp:160
NamesList nameList()
ElementsLocation::nameList.
Definition: elementslocation.cpp:532
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
Definition: qetproject.h:51
ElementsLocation copy() override
Definition: elementcollectionhandler.cpp:276
virtual ElementsLocation copy()=0
Definition: nameslist.h:30
The ECHSFileToFile class Manage the copy of directory or element from a file system collection to ano...
Definition: elementcollectionhandler.h:48
XmlElementCollection * projectCollection() const
ElementsLocation::projectCollection.
Definition: elementslocation.cpp:519
@ Erase
Erase the target content.
Definition: qet.h:130
ElementCollectionHandler()
ElementCollectionHandler::ElementCollectionHandler.
Definition: elementcollectionhandler.cpp:305
QET::Action selectedAction() const
Definition: renamedialog.h:37
The ECHSToXml class Manage the copy of a directory or element from a collection (no matter if the sou...
Definition: elementcollectionhandler.h:96
QDomElement toXml(QDomDocument &, const QHash< QString, QString > &=QHash< QString, QString >()) const
Definition: nameslist.cpp:169
The ECHSXmlToFile class Manage the copy of a directory or element from an xml collection to a file.
Definition: elementcollectionhandler.h:71
ECHSFileToFile(ElementsLocation &source, ElementsLocation &destination)
Definition: elementcollectionhandler.cpp:38