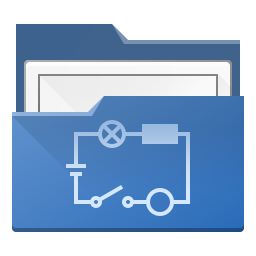 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef XMLPROJECTELEMENTCOLLECTIONITEM2_H
19 #define XMLPROJECTELEMENTCOLLECTIONITEM2_H
22 #include <QDomElement>
39 bool isDir()
const override;
42 QString
name()
const override;
51 bool hide_element =
false);
56 void populate(
bool set_data =
true,
bool hide_element =
false);
60 bool hide_element =
false);
67 #endif // XMLPROJECTELEMENTCOLLECTIONITEM2_H
QString localName() override
XmlProjectElementCollectionItem::localName.
Definition: xmlprojectelementcollectionitem.cpp:54
QList< QString > keys(KeyOrder=None) const
Definition: diagramcontext.cpp:49
XmlElementCollection * embeddedElementCollection() const
QETProject::embeddedCollection.
Definition: qetproject.cpp:236
QString title() const
Definition: qetproject.cpp:366
bool isElement() const override
XmlProjectElementCollectionItem::isElement.
Definition: xmlprojectelementcollectionitem.cpp:44
XmlProjectElementCollectionItem()
XmlProjectElementCollectionItem::XmlProjectElementCollectionItem Constructor.
Definition: xmlprojectelementcollectionitem.cpp:27
void populate(bool set_data=true, bool hide_element=false)
XmlProjectElementCollectionItem::populate Create the childs of this item.
Definition: xmlprojectelementcollectionitem.cpp:244
bool isDir() const override
XmlProjectElementCollectionItem::isDir.
Definition: xmlprojectelementcollectionitem.cpp:34
QString name() const override
XmlProjectElementCollectionItem::name.
Definition: xmlprojectelementcollectionitem.cpp:77
QString collectionPath() const override
XmlProjectElementCollectionItem::collectionPath.
Definition: xmlprojectelementcollectionitem.cpp:86
QETProject * project() const
XmlProjectElementCollectionItem::project.
Definition: xmlprojectelementcollectionitem.cpp:162
The ElementCollectionItem class This class represent a item (a directory or an element) in a element ...
Definition: elementcollectionitem.h:30
QDomElement m_dom_element
Definition: xmlprojectelementcollectionitem.h:64
void setXmlElement(const QDomElement &element, QETProject *project, bool set_data=true, bool hide_element=false)
XmlProjectElementCollectionItem::setXmlElement Set the managed content of this item.
Definition: xmlprojectelementcollectionitem.cpp:283
QIcon icon() const
ElementLocation::icon.
Definition: elementslocation.cpp:756
virtual QString embeddedPath() const
XmlProjectElementCollectionItem::embeddedPath.
Definition: xmlprojectelementcollectionitem.cpp:100
void setUpData() override
XmlProjectElementCollectionItem::setUpData SetUp the data of this item.
Definition: xmlprojectelementcollectionitem.cpp:191
int rowForInsertItem(const QString &name)
ElementCollectionItem::rowForInsertItem Return the row for insert a new child item to this item with ...
Definition: elementcollectionitem.cpp:116
void addChildAtPath(const QString &collection_name) override
XmlProjectElementCollectionItem::addChildAtPath Ask to this item item to add a new child with collect...
Definition: xmlprojectelementcollectionitem.cpp:136
QString projectCollectionPath() const
ElementsLocation::projectCollectionPath.
Definition: elementslocation.cpp:176
QDomElement root() const
XmlElementCollection::root The root is the first DOM-Element the xml collection, the tag name of the ...
Definition: xmlelementcollection.cpp:110
QIcon ProjectFileGP
Definition: qeticons.cpp:148
QIcon Folder
Definition: qeticons.cpp:94
@ Type
Definition: xmlprojectelementcollectionitem.h:36
QVariant value(const QString &key) const
Definition: diagramcontext.cpp:100
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
Definition: qetproject.h:51
void setProject(QETProject *project, bool set_data=true, bool hide_element=false)
XmlProjectElementCollectionItem::setProject Set the project for this item. Use this method for set th...
Definition: xmlprojectelementcollectionitem.cpp:175
QList< QDomElement > directories(const QDomElement &parent_element) const
XmlElementCollection::directories.
Definition: xmlelementcollection.cpp:203
bool isCollectionRoot() const override
XmlProjectElementCollectionItem::isCollectionRoot.
Definition: xmlprojectelementcollectionitem.cpp:120
QString name() const
ElementLocation::name.
Definition: elementslocation.cpp:776
The XmlProjectElementCollectionItem class This class specialise ElementCollectionItem for manage an x...
Definition: xmlprojectelementcollectionitem.h:32
QList< QDomElement > elements(const QDomElement &parent_element) const
XmlElementCollection::elements.
Definition: xmlelementcollection.cpp:247
DiagramContext elementInformations() const
ElementsLocation::elementInformations.
Definition: elementslocation.cpp:811
QETProject * m_project
Definition: xmlprojectelementcollectionitem.h:63
int type() const override
Definition: xmlprojectelementcollectionitem.h:37
QIcon tr
Definition: qeticons.cpp:206
Definition: diagramcontext.h:56
void setUpIcon() override
XmlProjectElementCollectionItem::setUpIcon SetUp the icon of this item. Because icon use several memo...
Definition: xmlprojectelementcollectionitem.cpp:223