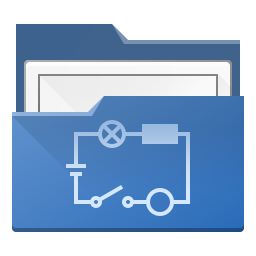 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ELEMENT_DIALOG_H
19 #define ELEMENT_DIALOG_H
24 class QDialogButtonBox;
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
ElementDialog(const ElementDialog &)
void loadCollections(bool common_collection, bool custom_collection, QList< QETProject * > projects)
ElementsCollectionModel::loadCollections Load the several collections in this model....
Definition: elementscollectionmodel.cpp:259
void indexClicked(const QModelIndex &index)
ElementDialog::indexClicked.
Definition: elementdialog.cpp:143
Definition: qfilenameedit.h:32
virtual QString collectionPath() const =0
The ElementCollectionItem class This class represent a item (a directory or an element) in a element ...
Definition: elementcollectionitem.h:30
@ SaveCategory
The dialog should select a category for saving.
Definition: elementdialog.h:44
@ OpenCategory
The dialog should open a category.
Definition: elementdialog.h:43
static ElementsLocation getOpenElementLocation(QWidget *parent=nullptr)
ElementDialog::getOpenElementLocation Display a dialog for open an element through her location.
Definition: elementdialog.cpp:280
bool isElement() const
ElementsLocation::isElement.
Definition: elementslocation.cpp:412
void setUpConnection()
ElementDialog::setUpConnection Setup connection of this dialog.
Definition: elementdialog.cpp:130
uint m_mode
Definition: elementdialog.h:66
QMessageBox::StandardButton question(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:53
QFileNameEdit * m_text_field
Definition: elementdialog.h:69
bool addToPath(const QString &)
ElementsLocation::addToPath Add a string to the actual path of this location.
Definition: elementslocation.cpp:320
bool isDirectory() const
ElementsLocation::isDirectory.
Definition: elementslocation.cpp:420
ElementsLocation m_location
Definition: elementdialog.h:67
ElementsCollectionModel * m_model
Definition: elementdialog.h:71
static QMap< uint, QETProject * > registeredProjects()
QETApp::registeredProjects.
Definition: qetapp.cpp:2353
static ElementsLocation execConfiguredDialog(int, QWidget *parent=nullptr)
ElementDialog::execConfiguredDialog launch a dialog with the chosen mode.
Definition: elementdialog.cpp:301
void checkCurrentLocation()
ElementDialog::checkCurrentLocation Update this dialog according to the current selected location and...
Definition: elementdialog.cpp:154
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
Definition: qetproject.h:51
ElementsLocation location() const
ElementDialog::location.
Definition: elementdialog.cpp:243
QTreeView * m_tree_view
Definition: elementdialog.h:70
Definition: elementdialog.h:34
bool isEmpty()
Definition: qfilenameedit.cpp:53
Definition: elementscollectionmodel.h:32
static ElementsLocation getSaveElementLocation(QWidget *parent=nullptr)
ElementDialog::getSaveElementLocation Display a dialog that allow to user to select an element (exist...
Definition: elementdialog.cpp:290
@ SaveElement
The dialog should select an element for saving.
Definition: elementdialog.h:42
QMessageBox::StandardButton critical(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:23
QDialogButtonBox * m_buttons_box
Definition: elementdialog.h:68
@ OpenElement
The dialog should open an element.
Definition: elementdialog.h:41
QIcon tr
Definition: qeticons.cpp:206
QIcon Cancel
Definition: qeticons.cpp:34
void setUpWidget()
ElementDialog::setUpWidget Build and setup the widgets of this dialog.
Definition: elementdialog.cpp:49
ElementDialog(uint=ElementDialog::OpenElement, QWidget *parent=nullptr)
ElementDialog::ElementDialog.
Definition: elementdialog.cpp:37
void checkAccept()
Definition: elementdialog.cpp:190