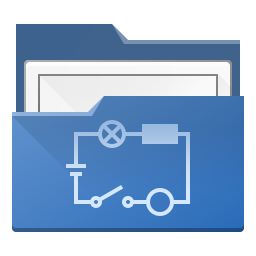 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef ITEMMODELCOMMAND_H
19 #define ITEMMODELCOMMAND_H
21 #include <QUndoCommand>
22 #include <QModelIndex>
25 class QAbstractItemModel;
34 ModelIndexCommand(QAbstractItemModel *model,
const QModelIndex &index, QUndoCommand *parent =
nullptr);
35 void setData(
const QVariant &value,
int role = Qt::DisplayRole);
37 virtual void redo()
override;
38 virtual void undo()
override;
56 void setData(
int section, Qt::Orientation orientation,
const QVariant &value,
int role = Qt::DisplayRole);
58 virtual void redo()
override;
59 virtual void undo()
override;
70 #endif // ITEMMODELCOMMAND_H
void setData(const QVariant &value, int role=Qt::DisplayRole)
ModelIndexCommand::setData.
Definition: itemmodelcommand.cpp:37
int m_role
Definition: itemmodelcommand.h:45
QPointer< QAbstractItemModel > m_model
Definition: itemmodelcommand.h:41
int m_role
Definition: itemmodelcommand.h:67
virtual void redo() override
ModelIndexCommand::redo Reimplemented from QUndoCommand.
Definition: itemmodelcommand.cpp:53
The ModelHeaderDataCommand class Change the data of a header.
Definition: itemmodelcommand.h:53
Qt::Orientation m_orientation
Definition: itemmodelcommand.h:63
QVariant m_new_value
Definition: itemmodelcommand.h:44
QVariant m_old_value
Definition: itemmodelcommand.h:64
The ModelIndexCommand class Change a data of an index of QAbstractItemModel.
Definition: itemmodelcommand.h:32
QModelIndex m_index
Definition: itemmodelcommand.h:42
virtual void redo() override
ModelHeaderDataCommand::redo Reimplemented from QUndoCommand.
Definition: itemmodelcommand.cpp:104
int m_section
Definition: itemmodelcommand.h:66
ModelHeaderDataCommand(QAbstractItemModel *model, QUndoCommand *parent=nullptr)
ModelHeaderDataCommand::ModelHeaderDataCommand.
Definition: itemmodelcommand.cpp:74
void setData(int section, Qt::Orientation orientation, const QVariant &value, int role=Qt::DisplayRole)
ModelHeaderDataCommand::setData See QAbstractItemModel::setHeaderData.
Definition: itemmodelcommand.cpp:87
QVariant m_new_value
Definition: itemmodelcommand.h:65
QPointer< QAbstractItemModel > m_model
Definition: itemmodelcommand.h:62
virtual void undo() override
ModelIndexCommand::undo Reimplemented from QUndoCommand.
Definition: itemmodelcommand.cpp:63
ModelIndexCommand(QAbstractItemModel *model, const QModelIndex &index, QUndoCommand *parent=nullptr)
ModelIndexCommand::ModelIndexCommand.
Definition: itemmodelcommand.cpp:26
QVariant m_old_value
Definition: itemmodelcommand.h:43
virtual void undo() override
ModelHeaderDataCommand::undo Reimplemented from QUndoCommand.
Definition: itemmodelcommand.cpp:115