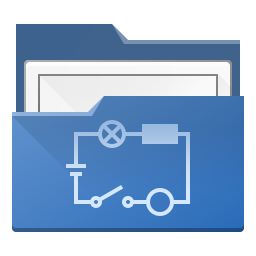 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef RECTANGLEEDITOR_H
19 #define RECTANGLEEDITOR_H
44 bool setParts(QList <CustomElementPart *> parts)
override;
71 Ui::RectangleEditor *
ui;
75 #endif // RECTANGLEEDITOR_H
void widthChanged()
Definition: rectangleeditor.cpp:243
void enableAnimation(bool animate=true)
QPropertyUndoCommand::enableAnimation True to enable animation.
Definition: qpropertyundocommand.cpp:92
QPointF editedTopLeft() const
RectangleEditor::topLeft.
Definition: rectangleeditor.cpp:127
QList< CustomElementPart * > currentParts() const override
Definition: styleeditor.cpp:543
QUndoStack & undoStack()
ElementScene::undoStack.
Definition: elementscene.cpp:603
PartRectangle * m_part
Definition: rectangleeditor.h:70
bool setParts(QList< CustomElementPart * > parts) override
Definition: rectangleeditor.cpp:101
void activeConnections(bool active)
RectangleEditor::activeConnections Enable/disable connection between editor widget and slot editingFi...
Definition: rectangleeditor.cpp:348
CustomElementPart * currentPart() const override
RectangleEditor::currentPart.
Definition: rectangleeditor.cpp:115
~RectangleEditor() override
RectangleEditor::~RectangleEditor.
Definition: rectangleeditor.cpp:45
qreal YRadius() const
Definition: partrectangle.h:70
Ui::RectangleEditor * ui
Definition: rectangleeditor.h:71
void heightChanged()
Definition: rectangleeditor.cpp:269
QList< QMetaObject::Connection > m_change_connections
Definition: rectangleeditor.h:72
QList< CustomElementPart * > currentParts() const override
Definition: rectangleeditor.cpp:119
Definition: styleeditor.h:35
Definition: elementitemeditor.h:34
bool m_locked
Definition: rectangleeditor.h:68
void xRadiusChanged()
Definition: rectangleeditor.cpp:295
void yRadiusChanged()
Definition: rectangleeditor.cpp:318
virtual ElementScene * elementScene() const
Definition: elementitemeditor.cpp:39
The RectangleEditor class This class provides a widget to edit rectangles within the element editor.
Definition: rectangleeditor.h:36
void xPosChanged()
Definition: rectangleeditor.cpp:191
qreal XRadius() const
Definition: partrectangle.h:68
void disconnectChangeConnections()
Definition: rectangleeditor.cpp:58
RectangleEditor(QETElementEditor *editor, PartRectangle *rect=nullptr, QWidget *parent=nullptr)
RectangleEditor::RectangleEditor.
Definition: rectangleeditor.cpp:32
QRectF rect
Definition: partrectangle.h:34
void setAnimated(bool animate=true, bool first_time=true)
QPropertyUndoCommand::setAnimated.
Definition: qpropertyundocommand.cpp:103
bool setPart(CustomElementPart *part) override
RectangleEditor::setPart.
Definition: rectangleeditor.cpp:71
Definition: autonumberingdockwidget.h:25
void editingFinished()
RectangleEditor::editingFinished Slot called when a editor widget is finish to be edited Update the g...
Definition: rectangleeditor.cpp:160
bool setParts(QList< CustomElementPart * >) override
StyleEditor::setParts Set several parts to edit by this editor. Note : editor can accept or refuse to...
Definition: styleeditor.cpp:510
void setUpChangeConnections()
setUpChangeConnections Setup the connection from the rectangles(s) to the widget, to update it when t...
Definition: rectangleeditor.cpp:49
Definition: partrectangle.h:31
The CustomElementPart class This abstract class represents a primitive of the visual representation o...
Definition: customelementpart.h:40
StyleEditor * m_style
Definition: rectangleeditor.h:69
void updateForm() override
RectangleEditor::updateForm.
Definition: rectangleeditor.cpp:134
void yPosChanged()
Definition: rectangleeditor.cpp:217
The QPropertyUndoCommand class This undo command manage QProperty of a QObject. This undo command can...
Definition: qpropertyundocommand.h:34
QIcon tr
Definition: qeticons.cpp:206
Definition: qetelementeditor.h:33
QVariant property(const char *name) const override
Definition: customelementgraphicpart.h:297