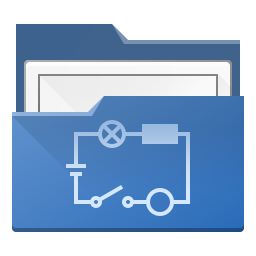 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef XMLELEMENTCOLLECTION_H
19 #define XMLELEMENTCOLLECTION_H
22 #include <QDomElement>
41 QDomElement
root()
const;
43 QDomNodeList
childs(
const QDomElement &parent_element)
const;
44 QDomElement
child(
const QDomElement &parent_element,
45 const QString &child_name)
const;
46 QDomElement
child(
const QString &path)
const;
48 const QDomElement &parent_element)
const;
50 const QDomElement &parent_element)
const;
52 const QDomElement &parent_element)
const;
54 const QDomElement &parent_element)
const;
55 QDomElement
element(
const QString &path)
const;
56 QDomElement
directory(
const QString &path)
const;
59 const QString &elmt_name,
60 const QDomElement &xml_definition);
64 const QString& rename = QString(),
65 bool deep_copy =
true);
66 bool exist (
const QString &path)
const;
73 QDomElement dom_element = QDomElement(),
84 const QString& rename = QString(),
85 bool deep_copy =
true);
88 const QString& rename = QString());
127 #endif // XMLELEMENTCOLLECTION_H
bool exist() const
ElementsLocation::exist.
Definition: elementslocation.cpp:472
bool createDir(const QString &path, const QString &name, const NamesList &name_list)
XmlElementCollection::createDir Create a child directorie at path path with the name name....
Definition: xmlelementcollection.cpp:587
QDomDocument m_dom_document
Definition: xmlelementcollection.h:123
void addName(const QString &, const QString &)
Definition: nameslist.cpp:43
ElementsLocation domToLocation(QDomElement dom_element) const
XmlElementCollection::domToLocation Return the element location who represent the xml element : dom_e...
Definition: xmlelementcollection.cpp:687
QString fileName() const
ElementLocation::fileName.
Definition: elementslocation.cpp:793
void directorieAdded(QString collection_path)
directorieAdded This signal is emited when a directorie is added to this collection
ElementsLocation copy(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString(), bool deep_copy=true)
XmlElementCollection::copy Copy the content represented by source (an element or a directory) to dest...
Definition: xmlelementcollection.cpp:545
QStringList directoriesNames(const QDomElement &parent_element) const
XmlElementCollection::directoriesNames.
Definition: xmlelementcollection.cpp:226
The XmlElementCollection class This class represent a collection of elements stored to xml.
Definition: xmlelementcollection.h:34
bool exist(const QString &path) const
XmlElementCollection::exist Return true if the path path exist in this collection.
Definition: xmlelementcollection.cpp:569
bool isFileSystem() const
ElementsLocation::isFileSystem.
Definition: elementslocation.cpp:428
void elementAdded(QString collection_path)
elementAdded This signal is emited when a element is added to this collection
QDomNodeList childs(const QDomElement &parent_element) const
XmlElementCollection::childs.
Definition: xmlelementcollection.cpp:128
QDomElement element(const QString &path) const
XmlElementCollection::element.
Definition: xmlelementcollection.cpp:291
void cleanUnusedElement()
XmlElementCollection::cleanUnusedElement Remove elements in this collection which is not used in the ...
Definition: xmlelementcollection.cpp:711
bool isElement() const
ElementsLocation::isElement.
Definition: elementslocation.cpp:412
QList< ElementsLocation > unusedElements() const
QETProject::unusedElements.
Definition: qetproject.cpp:1117
QStringList elementsNames(const QDomElement &parent_element) const
XmlElementCollection::elementsNames.
Definition: xmlelementcollection.cpp:269
ElementsLocation copyElement(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString())
XmlElementCollection::copyElement Copy the element represented by source to destination (must be a di...
Definition: xmlelementcollection.cpp:851
QString addElement(ElementsLocation &location)
XmlElementCollection::addElement Add the element at location to this collection. The element is copie...
Definition: xmlelementcollection.cpp:333
QDomElement fileSystemElementToXmlCollectionElement(QDomDocument &document, QFile &file, const QString &rename=QString())
Definition: qetxml.cpp:220
void directoryRemoved(QString collection_path)
directoryRemoved This signal is emited when a directory is removed to this collection
QDomElement xml() const
ElementsLocation::xml.
Definition: elementslocation.cpp:575
bool isDirectory() const
ElementsLocation::isDirectory.
Definition: elementslocation.cpp:420
bool addElementDefinition(const QString &dir_path, const QString &elmt_name, const QDomElement &xml_definition)
XmlElementCollection::addElementDefinition Add the élément defintion xml_definition in the directory ...
Definition: xmlelementcollection.cpp:477
QString projectCollectionPath() const
ElementsLocation::projectCollectionPath.
Definition: elementslocation.cpp:176
QDomElement child(const QDomElement &parent_element, const QString &child_name) const
XmlElementCollection::child If parent_element have child element with an attribute name = child_name,...
Definition: xmlelementcollection.cpp:148
QETProject * m_project
Definition: xmlelementcollection.h:124
QDomElement root() const
XmlElementCollection::root The root is the first DOM-Element the xml collection, the tag name of the ...
Definition: xmlelementcollection.cpp:110
QString fileSystemPath() const
ElementsLocation::fileSystemPath.
Definition: elementslocation.cpp:193
void elementRemoved(QString collection_path)
elementRemoved This signal is emited when an element is removed to this collection
bool isProject() const
ElementsLocation::isProject.
Definition: elementslocation.cpp:459
QDomElement directory(const QString &path) const
XmlElementCollection::directory.
Definition: xmlelementcollection.cpp:309
QString collectionPath(bool protocol=true) const
ElementsLocation::collectionPath Return the path of the represented element relative to collection if...
Definition: elementslocation.cpp:160
NamesList nameList()
ElementsLocation::nameList.
Definition: elementslocation.cpp:532
The ElementsLocation class This class represents the location, the location of an element or of a cat...
Definition: elementslocation.h:46
Definition: qetproject.h:51
Definition: nameslist.h:30
QList< QDomElement > directories(const QDomElement &parent_element) const
XmlElementCollection::directories.
Definition: xmlelementcollection.cpp:203
ElementsLocation copyDirectory(ElementsLocation &source, ElementsLocation &destination, const QString &rename=QString(), bool deep_copy=true)
XmlElementCollection::copyDirectory Copy the directory represented by source to destination....
Definition: xmlelementcollection.cpp:749
QDomElement fileSystemDirToXmlCollectionDir(QDomDocument &document, const QDir &dir, const QString &rename=QString())
Definition: qetxml.cpp:175
XmlElementCollection * projectCollection() const
ElementsLocation::projectCollection.
Definition: elementslocation.cpp:519
QDomElement importCategory() const
XmlElementCollection::importCategory.
Definition: xmlelementcollection.cpp:119
bool removeElement(const QString &path)
XmlElementCollection::removeElement Remove the element at path path.
Definition: xmlelementcollection.cpp:516
QList< QDomElement > elements(const QDomElement &parent_element) const
XmlElementCollection::elements.
Definition: xmlelementcollection.cpp:247
XmlElementCollection(QETProject *project)
XmlElementCollection::XmlElementCollection Build an empty collection. The collection start by :
Definition: xmlelementcollection.cpp:37
QETProject * project() const
ElementsLocation::project.
Definition: elementslocation.cpp:365
void cleanUnusedDirectory()
XmlElementCollection::cleanUnusedDirectory Remove the empty directories of this collection.
Definition: xmlelementcollection.cpp:721
QList< ElementsLocation > elementsLocation(QDomElement dom_element=QDomElement(), bool childs=true) const
XmlElementCollection::elementsLocation Return all locations stored in dom_element (element and direct...
Definition: xmlelementcollection.cpp:642
void setPath(const QString &path)
ElementsLocation::setPath Set the path of this item. The path can be relative to a collection (start ...
Definition: elementslocation.cpp:217
void elementChanged(QString collection_path)
elementChanged This signal is emited when the defintion of the element at path was changed
QDomElement toXml(QDomDocument &, const QHash< QString, QString > &=QHash< QString, QString >()) const
Definition: nameslist.cpp:169
bool removeDir(const QString &path)
XmlElementCollection::removeDir Remove the directory at path path.
Definition: xmlelementcollection.cpp:621