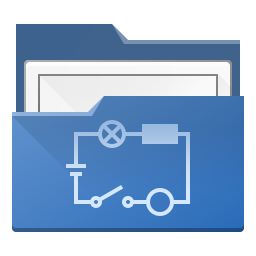 |
QElectroTech
0.8-dev
|
Go to the documentation of this file.
18 #ifndef TITLEBLOCK_SLASH_INTEGRATION_MOVE_TEMPLATES_HANDLER_H
19 #define TITLEBLOCK_SLASH_INTEGRATION_MOVE_TEMPLATES_HANDLER_H
QString nameForRenamingOperation() override
Definition: integrationmovetemplateshandler.cpp:98
void initDialog()
Definition: integrationmovetemplateshandler.cpp:145
@ Ignore
Skip the current item.
Definition: qet.h:129
Definition: integrationmovetemplateshandler.h:27
QDomElement getTemplateXmlDescription() const
Definition: templatelocation.cpp:138
~IntegrationMoveTitleBlockTemplatesHandler() override
Definition: integrationmovetemplateshandler.cpp:36
QDialog * integ_dialog_
Dialog in case of conflict when integrating a title block template.
Definition: integrationmovetemplateshandler.h:57
QET::Action errorWithATemplate(const TitleBlockTemplateLocation &, const QString &) override
Definition: integrationmovetemplateshandler.cpp:82
QDialogButtonBox * buttons_
Definition: integrationmovetemplateshandler.h:61
QRadioButton * use_existing_template_
Radio button the user may click to use the existing template and stop the integration.
Definition: integrationmovetemplateshandler.h:62
QRadioButton * erase_template_
Radio button the user may click for the integrated template to erase the existing one.
Definition: integrationmovetemplateshandler.h:64
@ Managed
the current item was handled by the Strategy object: do not treat it and continue
Definition: qet.h:132
QButtonGroup * button_group2_
Definition: integrationmovetemplateshandler.h:71
IntegrationMoveTitleBlockTemplatesHandler(QWidget *=nullptr)
Definition: integrationmovetemplateshandler.cpp:26
void correctRadioButtons()
Definition: integrationmovetemplateshandler.cpp:241
QRadioButton * integrate_new_template_
Radio button the user may click to integrate the template.
Definition: integrationmovetemplateshandler.h:63
IntegrationMoveTitleBlockTemplatesHandler(const IntegrationMoveTitleBlockTemplatesHandler &)
QRadioButton * integrate_both_
Definition: integrationmovetemplateshandler.h:69
Definition: movetemplateshandler.h:30
QString dateString() const
Definition: integrationmovetemplateshandler.cpp:105
@ Rename
the target has to be renamed
Definition: qet.h:133
Definition: templatelocation.h:29
QString toString() const
Definition: templatelocation.cpp:108
QString newNameForTemplate(const TitleBlockTemplateLocation &)
Definition: integrationmovetemplateshandler.cpp:114
@ Abort
abort the whole operation, ignoring the curent item
Definition: qet.h:131
QLabel * dialog_label_
Definition: integrationmovetemplateshandler.h:58
QET::Action askUser(const TitleBlockTemplateLocation &, const TitleBlockTemplateLocation &)
Definition: integrationmovetemplateshandler.cpp:124
QWidget * parent_widget_
Widget used as parent to display dialogs.
Definition: integrationmovetemplateshandler.h:55
void radioButtonleftMargin(QRadioButton *)
Definition: integrationmovetemplateshandler.cpp:234
Action
Definition: qet.h:127
QButtonGroup * button_group1_
Definition: integrationmovetemplateshandler.h:70
TitleBlockTemplatesCollection * parentCollection() const
Definition: templatelocation.cpp:57
@ Erase
Erase the target content.
Definition: qet.h:130
QVBoxLayout * dialog_vlayout_
Definition: integrationmovetemplateshandler.h:59
QET::Action templateAlreadyExists(const TitleBlockTemplateLocation &src, const TitleBlockTemplateLocation &dst) override
Definition: integrationmovetemplateshandler.cpp:44
Definition: templatescollection.h:34
QString rename_
Name to be used when renaming a title block template.
Definition: integrationmovetemplateshandler.h:56
QMessageBox::StandardButton critical(QWidget *, const QString &, const QString &, QMessageBox::StandardButtons=QMessageBox::Ok, QMessageBox::StandardButton=QMessageBox::NoButton)
Definition: qetmessagebox.cpp:23
QIcon tr
Definition: qeticons.cpp:206
QIcon Cancel
Definition: qeticons.cpp:34
QGridLayout * dialog_glayout
Definition: integrationmovetemplateshandler.h:60
QString name() const
Definition: templatelocation.cpp:73